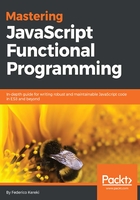
An unnecessary mistake
There is, however, a common (though in fact, harmless) mistake usually done. You often see code like this:
fetch("some/remote/url").then(function(data) {
processResult(data);
});
What does this code do? The idea is that a remote URL is fetched, and when the data arrives, a function is called -- and this function itself calls processResult with data as an argument. That is to say, in the then() part, we want a function that, given data, calculates processResult(data)... don't we already have such a function?
A small bit of theory: In lambda calculus terms, we are replacing λx.func x by simply a function -- this is called an eta conversion, more specifically an eta reduction. (If you were to do it the other way round, it would be an eta abstraction.) In our case, it could be considered a (very, very small!) optimization, but its main advantage is shorter, more compact code.
Basically, the rule we can apply is that whenever you see something like the following:
function someFunction(someData) {
return someOtherFunction(someData);
}
You may replace it with just someOtherFunction. So, in our example, we can directly write what follows:
fetch("some/remote/url").then(processResult);
This code is exactly equivalent to the previous way (or, infinitesimally quicker, since you avoid one function call) but simpler to understand... or not?
This programming style is called pointfree style or tacit style, and its main characteristic is that you never specify the arguments for each function application. An advantage of this way of coding, is that it helps the writer (and the future readers of the code) think about the functions themselves and their meanings, instead of working at low level, passing data around and working with it. In the shorter version of the code, there are no extraneous or irrelevant details: if you understand what the called function does, then you understand the meaning of the complete piece of code. In our text, we'll often (but not necessarily always) work in this way.
Unix/Linux users may already be accustomed to this style, because they work in a similar way when they use pipes to pass the result of a command as an input to another. When you write something as ls | grep doc | sort the output of ls is the input to grep, and the latter's output is the input to sort -- but input arguments aren't written out anywhere; they are implied. We'll come back to this in the PointFree Style section of Chapter 8, Connecting Functions - Pipelining and Composition.