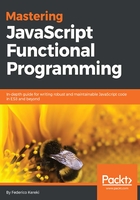
A React+Redux reducer
We can see another example that involves assigning functions. As we mentioned earlier in this chapter, React+Redux works by dispatching actions that are processed by a reducer. Usually, the reducer includes code with a switch:
function doAction(state = initialState, action) {
let newState = {};
switch (action.type) {
case "CREATE":
// update state, generating newState,
// depending on the action data
// to create a new item
return newState;
case "DELETE":
// update state, generating newState,
// after deleting an item
return newState;
case "UPDATE":
// update an item,
// and generate an updated state
return newState;
default:
return state;
}
}
Providing initialState as a default value for state is a simple way of initializing the global state the first time around. Pay no attention to that default; it's not relevant for our example, and I included it just for completeness.
By taking advantage of the possibility of storing functions, we can build a dispatch table and simplify the preceding code. First, we would initialize an object with the code for the functions for each action type. Basically, we are just taking the preceding code, and creating separate functions:
const dispatchTable = {
CREATE: (state, action) => {
// update state, generating newState,
// depending on the action data
// to create a new item
return newState;
},
DELETE: (state, action) => {
// update state, generating newState,
// after deleting an item
return newState;
},
UPDATE: (state, action) => {
// update an item,
// and generate an updated state
return newState;
}
};
We have stored the different functions that process each type of action, as attributes in an object that will work as a dispatcher table. This object is created only once and is constant during the execution of the application. With it, we can now rewrite the action processing code in a single line of code:
function doAction2(state = initialState, action) {
return dispatchTable[action.type]
? dispatchTable[action.type](state, action)
: state;
}
Let's analyze it: given the action, if action.type matches an attribute in the dispatching object, we execute the corresponding function, taken from the object where it was stored. If there isn't a match, we just return the current state, as Redux requires. This kind of code wouldn't be possible if we couldn't handle functions (storing and recalling them) as first-class objects.