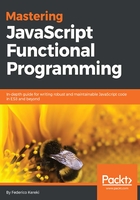
Working with methods
There is, however, a case you should be aware of: what happens if you are calling an object's method? If your original code had been something along the lines of:
fetch("some/remote/url").then(function(data) {
myObject.store(data);
});
Then the seemingly obvious transformed code would fail:
fetch("some/remote/url").then(myObject.store);
Why? The reason is that in the original code, the called method is bound to an object (myObject) but in the modified code, it isn't bound, and it is just a free function. We can then fix it in a simple way by using bind() as:
fetch("some/remote/url").then(myObject.store.bind(myObject));
This is a general solution. When dealing with a method, you cannot just assign it; you must use .bind( so the correct context will be available. Code like:
function doSomeMethod(someData) {
return someObject.someMethod(someData);
}
Should be converted to:
const doSomeMethod = someObject.someMethod.bind(someObject);
Read more on .bind() at https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_objects/Function/bind.
This looks rather awkward, and not too elegant, but it's required so the method will be associated to the correct object. We will see one application of this when we promisify functions in Chapter 6, Producing Functions - Higher-Order Functions. Even if this code isn't so nice to look at, whenever you have to work with objects (and, remember, we didn't say that we would be trying to aim for fully FP code and that we would accept other constructs if they made things easier) you'll have to remember to bind methods before passing them as first-class objects, in pointfree style.