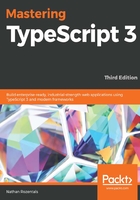
Arrays
Besides the basic JavaScript types of string, number, and boolean, TypeScript also has two other basic data types that we will now take a closer look at—arrays and enums. Let's look at the syntax for defining arrays.
An array is simply marked with the [] notation, similar to JavaScript, and each array can be strongly typed to hold values of a specific type, as seen in the following code:
var arrayOfNumbers: number [] = [1,2,3]; arrayOfNumbers = [3,4,5,6,7,8,9]; console.log(`arrayOfNumbers: ${arrayOfNumbers}`); arrayOfNumbers = ["1", "2", "3"];
Here, we start by defining an array named arrayOfNumbers, and further specifying that each element of this array must be of type number. We then reassign this array to hold some different numerical values. Interestingly enough, we are able to assign any number of elements to an array, as long as each element is of the correct type. We then use a simple template string to print the value of the array to the console.
The last line of this snippet, however, will generate the following error message:
error TS2322: Type 'string[]' is not assignable to type 'number[]'.
This error message is warning us that the variable arrayOfNumbers is strongly typed to only accept values of the type number. As our code is trying to assign an array of strings to this array of numbers, an error is generated. The output of this code snippet is as follows:
arrayOfNumbers: 3,4,5,6,7,8,9