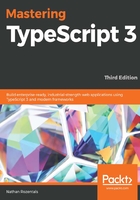
Template strings
Before we continue with our discussion on types, let's explore a simple method for logging the value of any variable to the console. It is worth noting that TypeScript allows for ES6 template string syntax, which is a convenient syntax for injecting values into strings. Consider the following code:
var myVariable = "test"; console.log("myVariable=" + myVariable);
Here, we are simply assigning a value to a variable, and then logging the result to the console. Note that the console.log function uses a little bit of formatting to make the message readable, by concatenating the strings with the "string" + variable syntax. Let's now take a look at the equivalent TypeScript code that uses the ES6 template string syntax:
var myVariable = "test"; console.log(`myVariable=${myVariable}`);
Here, on the second line of this code snippet, we are logging some information to the console, and are using the template string syntax. There are two important things to note here. Firstly, we have switched the string definition from a double quote (") to a backtick (`). Using a backtick signals to the TypeScript compiler that it should look for template values within the string enclosed by the backticks, and replace them with actual values. Secondly, we have used a special ${ ... } syntax within the string to denote a template. TypeScript will inject the value of any variable that is currently in scope into the string for us. This is a convenient method of dealing with string formatting.
In the remainder of this chapter, we will use this string template syntax whenever we log values to the console.
Another handy way of using this template string syntax is when we need to log an entire complex object, including all of its properties, to the console. Consider the following code:
var complexObject = { id: 2, name: 'testObject' } console.log(`complexObject = ${JSON.stringify(complexObject)}`);
Here, we define an object named complexObject that has an id and a name property. We are then using template string syntax to log the value of this object to the console. But in this example, we are calling the JSON.stringify function, and passing in the complexObject variable as a parameter. This means that anything within the ${ ... } is not limited to simple types, but can be valid TypeScript. The output of this code is as follows:
complexObject = {"id":2,"name":"testObject"}
Here, we can see that the result of calling the JSON.stringify function is a string representation of the entire object, which we are logging to the console.