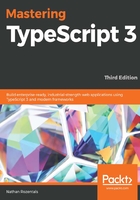
for...in and for...of
When working with arrays, it is common practice to loop through array items in order to perform a task. This is generally accomplished within a for loop by manipulating an array index, as shown in the following code:
var arrayOfStrings : string[] = ["first", "second", "third"]; for( var i = 0; i < arrayOfStrings.length; i++ ) { console.log(`arrayOfStrings[${i}] = ${arrayOfStrings[i]}`); }
Here, we have an array named arrayOfStrings, and a standard for loop that is using the variable i as an index into our array. We access the array item using the syntax arrayOfStrings[i]. The output of this code is as follows:
arrayOfStrings[0] = first arrayOfStrings[1] = second arrayOfStrings[2] = third
TypeScript can also use the ES6 for...in syntax to simplify looping through arrays. Here is an example of the previous for loop expressed using this new syntax:
for( var itemKey in arrayOfStrings) { var itemValue = arrayOfStrings[itemKey]; console.log(`arrayOfStrings[${itemKey}] = ${itemValue}`); }
Here, we have simplified the for loop by using the itemKey in arrayOfStrings syntax. Note that the value of the variable itemKey will iterate through the keys of the array, and not the array elements themselves. Within the for loop, we are first de-referencing the array to extract the array value for this itemKey, and then logging both the itemKey and the itemValue to the console. The output of this code is as follows:
arrayOfStrings[0] = first arrayOfStrings[1] = second arrayOfStrings[2] = third
If we do not necessarily need to know the keys of the array and are simply interested in the values held within the array, we can further simplify looping through arrays using the for...of syntax. Consider the following code:
for( var arrayItem of arrayOfStrings ) { console.log(`arrayItem = ${arrayItem} `); }
Here, we are using the for...of syntax to iterate over each value of the arrayOfStrings array. Each time that the for loop is executed, the arrayItem variable will hold the next element in the array. The output of this code is as follows:
arrayItem = first arrayItem = second arrayItem = third