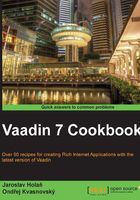
Creating an adjustable layout using split panels
In a more complex layout, it is better to let the user adjust it. If we want to use a more flexible layout, we can use split panels. This recipe is about creating a complex layout with different components, for example, menu, editor, properties view, and Help view.

How to do it...
Carry out the following steps to create an adjustable layout:
- We create a simple Vaadin project with the main UI class called, for example,
Demo
:public class Demo extends UI {…}
- Our application will be based on split panels. As a main panel, we use
HorizontaSplitPanel
. This panel is divided into two areas. The first is on the left side, the second is on right the side.public class AdjustableLayout extends HorizontalSplitPanel {…}
- In the constructor, we set the properties of this main panel. On the left side we insert the main menu, on the right side we insert the other content. The left area will take 10 percent of the whole panel. And with the
setSizeFull()
method, we set height and width to 100 percent of the window.public AdjustableLayout() { setFirstComponent(createMenu()); setSecondComponent(createContentPanel()); setSplitPosition(10, Unit.PERCENTAGE); setSizeFull(); }
- Now, we can continue with the
createMenu()
method. For our example, we will just create a simple menu based onTree
. This menu does not have any special logic. We need it only to fill the space.private Tree createMenu() { Tree menu = new Tree(); for (int i = 1; i < 6; i++) { String item = "item" + i; String childItem = "subitem" + i; menu.addItem(item); menu.addItem(childItem); menu.setParent(childItem, item); menu.setChildrenAllowed(childItem, false); } return menu; }
- In the
createContentPanel()
method, we will add other split panels. This time it isVerticalSplitPanel
. The first area is on the top and the second is on the bottom of the panel. On the top we insert an editor panel and on the bottom we insert a table. For editor panel we set aside 80 percent of the area.private Component createContentPanel(){ VerticalSplitPanel contentPanel = new VerticalSplitPanel(); contentPanel.setFirstComponent(createEditorPanel()); contentPanel.setSecondComponent(createTable()); contentPanel.setSplitPosition(80, Unit.PERCENTAGE); return contentPanel; }
- For the editor panel, we again use
HorizontalSplitPanel
. On the left side we insert a rich text area and on the right side we insert a label. Editor takes 80 percent of the panel. When we work with raw HTML code in a web application, it's important to be very sure that the HTML is safe and no malicious code is injected here. For this reason, we will use theSafeHtml
interface. An object that implements this interface encapsulates HTML that is guaranteed to be safe to use (with respect to potential cross-site scripting vulnerabilities) in an HTML context.private Component createEditorPanel() { SafeHtml safeHtml = SafeHtmlUtils.fromSafeConstant( "<b>Help</b> <br />" + LIPSUM); HorizontalSplitPanel editorPanel = new HorizontalSplitPanel(); RichTextArea editor = new RichTextArea(); editor.setSizeFull(); editor.setValue(LIPSUM); editorPanel.setFirstComponent(editor); editorPanel.setSecondComponent( new Label(safeHtml.asString(), ContentMode.HTML)); editorPanel.setSplitPosition(80, Unit.PERCENTAGE); return editorPanel; }
- Now we need some text for the rich text and label area. We create a constant lipsum which contains a simple string of some words called Lorem Ipsum, for example, generated from http://www.lipsum.com/.
private static final String LIPSUM = "Lorem ipsum dolor …";
- We will fill the bottom of the panel with a table. In a lot of cases it can be the table of properties. The size of the table is set to full. It means that this component takes the whole area.
private Table createTable() { Table table = new Table(); table.addContainerProperty("Name", String.class, null); table.addContainerProperty("Value", String.class, null); table.addItem(new Object[] { "Color", "blue" }, null); table.addItem(new Object[] { "Height", "600 px" }, null); table.addItem(new Object[] { "Width", "1024 px" }, null); table.setSizeFull(); return table; }
- Now we can use our layout in the root application. We add an instance of
AdjustableLayout
to theinit()
method.public class Demo extends UI { @Override public void init(VaadinRequest request) { setContent(new AdjustableLayout()); } }
How it works...
Users can adjust the layout by dragging the bar with the mouse. To disable changing the bar position, we can use the setLocked(true)
method. With the setSplitPosition(float position)
method, we can programmatically set the bar position. When we don't set any unit, previous unit will be used. SplitPanel
only supports pixel or percent from the enumeration unit in the interface. This value position means how big an area the first component takes of the panel. The second area takes the rest. If we want to define primarily the size of the second area, we set the reverse parameter to true
.
For example, take a look at the following line of code:
setSplitPosition(30, Unit.Pixels, true);
This means that second area takes 30 pixels and first takes the rest.
For setting the size, we can also use the method that takes a string, for example:
setWidth("25%");
See also
- For more information about the Application Program Interface (API) of the
VerticalSplitPanel
class, visit https://vaadin.com/api/7.0.0/com/vaadin/ui/VerticalSplitPanel.html - For more information about the API of the
HorizontalSplitPanel
class visit https://vaadin.com/api/7.0.0/com/vaadin/ui/HorizontalSplitPanel.html - The Developer's Guide about
SafeHtml
is available at https://developers.google.com/web-toolkit/doc/latest/DevGuideSecuritySafeHtml