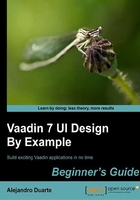
Time for action – using an InlineDateField component
Let's change the DateField
example a little bit:
- Open or import the datefield example in your IDE.
- Replace
DateField
withInlineDateField
inDateFieldUI.java
. - Deploy and run the example.
What just happened?
This is the result you get:

You cannot type the year now, you have to select it from the component.
Uploading files
Study this simple example that displays (in the console) the content of a text file uploaded by the user:
public class UploadUI extends UI implements Receiver { @Override protected void init(VaadinRequest request) { Upload upload = new Upload( "Select a text file and look at the console", this); VerticalLayout layout = new VerticalLayout(); layout.addComponent(upload); setContent(layout); } @Override public OutputStream receiveUpload(String filename, String mimeType) { return new OutputStream() { @Override public void write(int b) throws IOException { System.out.print((char) b); } }; } }
The Upload
class displays a native (browser-dependent) button to select a file and a Vaadin button to upload the file to the server:

We are passing a Receiver
interface (implemented in our UploadUI
class) to process the upload byte-by-byte. The receiveUpload
method must return an OutputStream
class that writes the content of the file being uploaded. In this example, we are taking each byte and printing it on the console, but you can do with the bytes whatever you want to. For example, you could write them to a file in the hard disk or in a BLOB in an SQL database.
No, really, how can we actually write the file to the server? OK, OK, here is a possible implementation of recieveUpload
that does the job:
@Override public OutputStream receiveUpload(String filename, String mimeType) { FileOutputStream output = null; try { output = new FileOutputStream("C:\\Users\\Alejandro\\" + filename); } catch (FileNotFoundException e) { e.printStackTrace(); } return output; }
Pop quiz – thinking in Vaadin
Check your knowledge:
Q1. Which method allows you to add options in a ComboBox
?
addOption(String option)
.addItem(String itemId)
.addItem(Object itemId)
.
Q2. If you want to execute some code when the user changes the value in a Field
component you must add a:
ValueChangeListener
.ClickListener
.ValueChangeEvent
.
Q3. To add a tooltip, you use the method:
setTooltip(String text)
.addTooltip(String text
).setDescription(String text)
.
Q4. You can add an error message to a Component
by calling:
setErrorMessage(String error)
.setComponentError(ErrorMessage errorMessage)
.
Q5. All input components implement (directly or indirectly):
Field
.Property
.- All of the above.
Q6. A Property
is:
- A value of a certain type.
- A collection of items.