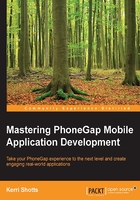
Managing version numbers
Although we've set up our copy-config
and copy-code
tasks to substitute the version number whenever {{{VERSION}}}
is encountered, we don't have any tasks that actually change the version. We could just edit package.json
, of course. But this is tedious and it can't be included automatically in any other Gulp task. Instead, let's use the gulp-bump
plugin to take care of this for us.
gulp-bump
is a very simple plugin: it is designed to take a package.json
(or similar) file and edit the version
property based on specific commands. Most versions are of the major.minor.patch
form and we can ask it to increment any portion by one. If you wanted, you could increment the patch
portion of the version to automatically track build numbers, for example.
Doing this is pretty simple. Let's first create another utility file, this time called gulp/utils/bump.js
:
var gulp = require("gulp"); var bump = require("gulp-bump"); module.exports = function bump(importance) { return gulp.src([path.join(process.cwd(), "package.json")]) .pipe(bump({type: importance})) .pipe(gulp.dest(process.cwd())); }
The importance
variable can be one of the following strings: major
, minor
, or patch
. Next, let's create three tasks that will allow us to call this method directly (again, these are in three separate files, indicated in the comment):
// gulp/tasks/version-bump-patch.js var bump = require("../utils/bump"); module.exports = { task: bump.bind(null, "patch") } // gulp/tasks/version-bump-minor.js var bump = require("../utils/bump"); module.exports = { task: bump.bind(null, "minor") } // gulp/tasks/version-bump-major.js var bump = require("../utils/bump"); module.exports = { task: bump.bind(null, "major") }
Now you can directly bump the version number by executing gulp version-bump-patch
. This, however, only edits package.json
. If you want the files in build/
to reflect this, you will need to also execute gulp copy
(or build
and so on).