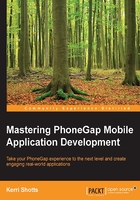
Copying assets
Now that we've created the basic Gulp configuration structure, let's create our first task to copy our app's assets from the source path to the destination path.
We can call this file gulp/tasks/copy-assets.js
, and it should look like this:
var merge = require("merge-stream"), gulp = require("gulp"), config = require("../config"), paths = require("../utils/paths"); function copyAssets() { return merge.apply(merge, config.assets.copy.map(function(asset) { var fqSourcePath = paths.makeFullPath(asset.src, paths.SRC); var fqTargetPath = paths.makeFullPath(asset.dest, paths.DEST); return gulp.src([fqSourcePath]) .pipe(gulp.dest(fqTargetPath)); }); } module.exports = { task: copyAssets }
The method, copyAssets
simply copies a lot of files based upon the project's file structure as specified in gulp/config.js
. The code here could be simpler, but you may find that you need to change which files need and don't need substitutions later. So, we've made it configurable. All you need to do is to change the files and destinations within config.assets.copy
in gulp/config.js
and this task will react accordingly.
Let's go over what this task is really doing:
- We're using our utility method
paths.makeFullPath
(which usespath.join
) to ensure that our configuration works across multiple platforms. On Unix-like systems, the path separator is/
; but on Windows systems, the path separator is actually\
. In order to simplify the configuration, however, we're using/
inconfig.assets.copy
.makeFullPathsplits
(/
)each one of the strings into arrays, and usespath.join
(which knows the correct path separator) to create the final path. map
iterates over an array and returns a new array using a given transformation. For example,[1, 2, 3].map(function(x) {return x*2;})
will return a new array of[2, 4, 6]
. In our case, we're returning an array ofgulp.src(…).pipe(gulp.dest(…))
chains. We can thenapply
the array tomerge
in order to combine all the tasks together.apply
is a way to call a function that accepts multiple arguments using an array instead. For example,console.log.apply(console,[1,2,3])
will log1 2 3
. This is different fromconsole.log([1,2,3])
which instead will log[1, 2, 3]
.
At this point, you can type the following on the command line and copy the project assets from their source location to their destination:
$ gulp copy-assets