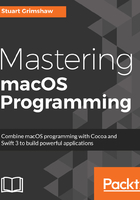
Functions
In this chapter, we will look at the basics of function declaration in Swift, and we'll leave the more advanced features until Chapter 5, Advanced Swift.
To define a function in Swift, we use the following syntax:
func functionName(argument: argumentType) -> returnType
{
// code
}
We can define a function that takes no arguments and returns no value as follows:
func printDate()
{
print(Date())
}
Note that it is not necessary to declare a Void return value, though we may do so if we choose.
We would call that function as follows:
printDate()
Arguments to the function are supplied in the parentheses following the function name, in the form argName: argType; if there are more than one, they are separated by commas:
func printPoint(x: Int, y:Int)
{
print(x, y)
}
We would call that function as follows:
printPoint(x: 11, y: 59)
The argument value(s) must be preceded by the argument name(s), as can be seen previously.
This is how we define a return value:
func increment(x: Int) -> Int
{
return x + 1
}
Calling this function looks as follows:
let y = increment(x: 0)
A function can have one (and only one) variadic argument, an arbitrary number of arguments of the same type, as well as other "normal" arguments:
func printMyInts(args: Int..., label: String)
{
print(label)
for x in args
{
print(x)
}
}
The variadic arguments are separated by commas in the call to the function:
printMyInts(args: 1, 1, 2, 3, 5, 0, label: "Fibonnacci Five-0")
It is quite simple to return any number of values, without having to resort to arrays, or dictionaries, or some other complex data structure, by using a tuple to enclose the return values:
func sumAndDifference(x: Int, y: Int) -> (sum: Int, diff: Int)
{
let sum = x + y
let difference = abs(x - y)
return (sum, difference)
}
We can access the results together as a tuple:
let results = sumAndDifference(x: 3, y: 5)
These can then be decomposed by index or argument name, as with any tuple:
print(results.0)
print(results.1)
print(results.sum)
print(results.diff)
But we can also do that in one step:
let (sum, difference) = sumAndDifference(x: 5, y: 8)
So far, so good; we have covered the basics of functions in Swift. But most of the good stuff is yet to come.