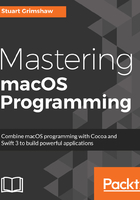
Enumerations
Swift offers another valuable structure, the Enum. While enumerations are a common feature of programming languages, Swift's Enum offers very much more than a simple mapping of integer values to labels.
Firstly, in cases where there is no logical mapping of Enum values to integers (or values of any other type), we can declare an Enum to be a type in its own right:
enum Color
{
case red
case amber
case green
}
So, here we have a case in which it would be nonsensical to map colors to integers. Swift will not allow us to try to derive some integer value from Color.red, either.
Note that the Enum name is capitalized, whereas a case is written in lowercase.
There are, however, frequent uses for an Enum that do correspond to some underlying value, and Swift lets us do this, too:
enum Medal: Int
{
case gold = 1
case silver
case bronze
}
Clearly, there is a correspondence between medals and positions, and so it makes absolute sense to map each Medal case to an Int. Note that, after we have set the Int value of the first case, the subsequent cases are assigned incremental values automatically (although there is nothing to stop you overriding this behavior with different values).
To access the underlying value of an Enum case, we use its rawValue property:
let position = Medal.bronze.rawValue
In the preceding code, the position constant is set to be equal to 3.
We can also use raw values to create a Medal as follows:
let myMedal = Medal(rawValue: 2)
myMedal, as you would expect, has the value Medal.silver. Using raw values like this makes comparison of Enum cases very simple:
Medal.gold.rawValue > Medal.silver.rawValue
The preceding line of code evaluates to false (did it catch you out?).
When the type of a variable or constant is already set, we can use an abbreviated syntax without the Enum name:
var nextMedal: Medal
nextMedal = .gold
Enums have also established themselves as a convenient way to express compile-time constants:
enum APIUrlStr: String
{
case uat = "http.www.theFirm.com/api/uat"
case prod = "http.www.theFirm.com/api"
}
By the way, Swift will turn Enum case names into Strings when the Enum itself is declared to map to Strings:
enum Eggs: String
{
case fried, scrambled, poached
}
let bestWayToEatEggs: String = Eggs.poached.rawValue
We'll leave Enums there for the time being; in Chapter 5, Advanced Swift, we will see that we have only just scratched the surface of Swift's Enum.