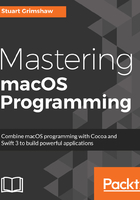
Tuples
We have seen already that the Set object's insert method returns a tuple (see Sets in this chapter); a tuple is a great way to pass around a lightweight, ad hoc data structure, one that may consist of mixed types, since you are not limited by the variety or number of different types:
let t1 = (1, 1.1, "1")
let t2 = (9, 9, 9)
let t3 = (t1, t2)
Tuples can contain any combination of types, including nested tuple. Like struct, tuple are passed by value.
Decomposition means accessing the individual values of a tuple, using its .index notation:
let i1 = t1.0
let f1 = t1.1
If it is convenient to name the elements of a tuple for improved clarity, we can do so:
let complex = (r: 11.5, i: 20.0, label: "startPoint")
let real = complex.r
let imaginary = complex.i
let label = complex.label
The preceding example may suggest the use of a typealias:
typealias complexNumber = (Double, Double, String)
var anotherComplex: complexNumber
Swift's type safety will now prevent us from creating a complexNumber object (or initializing our anotherComplex variable) with the wrong value types.
We can't do much more with tuple objects than this, so they are no substitute for full-fledged class and struct structures, but they are a very useful quick-and-easy way to, for example, create a function that returns more than one value, and are an essential part of our data-structure toolkit.