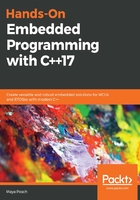
Templates
It's often thought that templates in C++ are very heavy, and carry a severe penalty for using them. This completely misses the point of templates, which is that templates are merely meant to be used as a shorthand method for automating the generation of nearly identical code from a single template – hence the name.
What this effectively means is that for any function or class template we define, the compiler will generate an inline implementation of the template each time the template is referenced.
This is a pattern we commonly see in the C++ standard template library (STL), which, as the name suggests, makes heavy use of templates. Take, for example, a data structure like a humble map:
std::map<std::string, int> myMap;
What happens here is that the singular template for an std::map is taken by the compiler, along with the template parameters we provide within the sharp brackets, filling in the template and writing an inline implementation in its spot.
Effectively, we get the same implementation as if we had written the entire data structure implementation by hand just for those two types. Since the alternative would be to write every implementation by hand for every conceivable built-in type and additional user-defined type, the use of a generic template saves us a lot of time, without sacrificing performance.