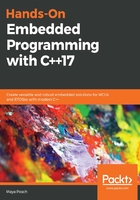
Type conversions
A type conversion occurs whenever a value is assigned to a compatible variable, which is not the exact same type as the value. Whenever a rule for conversion exists, this conversion can be done implicitly, otherwise an explicit hint (cast) can be provided to the compiler to invoke a specific rule where ambiguity exists.
Whereas C only has implicit and explicit type casting, C++ expands on this with a number of template-based functions, allowing you to cast both regular types and objects (classes) in a variety of ways:
- dynamic_cast <new_type> (expression)
- reinterpret_cast <new_type> (expression)
- static_cast <new_type> (expression)
- const_cast <new_type> (expression)
Here, dynamic_cast guarantees that the resulting object is valid, relying on runtime type information (RTTI) (see the later section on it) for this. A static_cast is similar, but does not validate the resulting object.
Next, reinterpret_cast can cast anything to anything, even unrelated classes. Whether this conversion makes sense is left to the developer, much like with a regular explicit conversion.
Finally, a const_cast is interesting in that it either sets or removes the const status of a value, which can be useful when you need a non-const version of a value for just one function. This does, however, also circumvent the type safety system and should be used very cautiously.