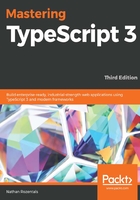
Optional parameters
When we call a JavaScript function that is expecting parameters, and we do not supply these parameters, then the value of the parameter within the function will be undefined. As an example of this, consider the following JavaScript code:
var concatStrings = function(a,b,c) { return a + b + c; } var concatAbc = concatStrings("a", "b", "c"); console.log("concatAbc :" + concatAbc); var concatAb = concatStrings("a", "b"); console.log("concatAb :" + concatAb);
The output of this code is as follows:
concatAbc :abc concatAb :abundefined
Here, we have defined a function called concatStrings that takes three parameters, a, b, and c, and simply returns the sum of these values. We are then calling this function with three arguments, and assigning the result to the variable concatAbc. As can be seen from the output, this returns the string "abc" as expected. If, however, we only supply two arguments, as seen with the usage of the concatAb variable, the function returns the string "abundefined". In JavaScript, if we call a function and do not supply a parameter, then the missing parameter will be undefined, which in this case is the parameter c.
TypeScript introduces the question mark ? syntax to indicate optional parameters. This allows us to mimic JavaScript calling syntax, where we can call the same function with some missing arguments. As an example of this, consider the following TypeScript code:
function concatStrings( a: string, b: string, c?: string) { return a + b + c; } var concat3strings = concatStrings("a", "b", "c"); console.log(`concat3strings : ${concat3strings}`); var concat2strings = concatStrings("a", "b"); console.log(`concat2strings : ${concat2strings}`); var concat1string = concatStrings("a");
Here, we have a strongly typed version of the original concatStrings JavaScript function that we were using previously. Note the addition of the ? character in the syntax for the third parameter: c?: string. This indicates that the third parameter is optional, and therefore, all of the preceding code will compile cleanly, except for the last line. The last line will generate an error:
error TS2554: Expected 2-3 arguments, but got 1
This error is generated because we are attempting to call the concatStrings function with only a single parameter. Our function definition, though, requires at least two parameters, with only the third parameter being optional.