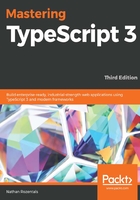
Anonymous functions
The JavaScript language also has the concept of anonymous functions. These are functions that are defined on the fly and don't specify a function name. Consider the following JavaScript code:
var addVar = function(a,b) { return a + b; } var addVarResult = addVar(2,3); console.log("addVarResult:"" + addVarResult);
Here, we define a function that has no name and adds two values. Because the function does not have a name, it is known as an anonymous function. This anonymous function is then assigned to a variable named addVar. The addVar variable can then be invoked as a function with two parameters, and the return value will be the result of executing the anonymous function. The output of this code will be as follows:
addVarResult:5
Let's now rewrite the preceding anonymous JavaScript function in TypeScript, as follows:
var addFunction = function(a:number, b:number) : number { return a + b; } var addFunctionResult = addFunction(2,3); console.log(`addFunctionResult : ${addFunctionResult}`);
Here, we see that TypeScript allows anonymous functions in the same way that JavaScript does, but also allows standard type annotations. The output of this TypeScript code is as follows:
addFunctionResult : 5