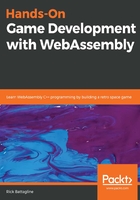
Rendering a texture to the HTML5 canvas
After we load a font from the virtual filesystem and then render that font to the texture, we need to take that texture and copy it to a location in our renderer object. After we have done that, we will need to take that renderer and present its contents to the HTML5 canvas element.
The following is the source code that renders the texture to the canvas:
SDL_QueryTexture( texture,
NULL, NULL,
&dest.w, &dest.h ); // query the width and height
dest.x -= dest.w / 2;
dest.y -= dest.h / 2;
SDL_RenderCopy( renderer, texture, NULL, &dest );
SDL_RenderPresent( renderer );
The call to the SDL_QueryTexture function is used to retrieve the width and height of the texture. We need to use these values in the destination rectangle so that we render our texture to the canvas without changing its dimensions. After that call, the program knows the width and height of the texture, so it can use those values to modify the x and y variables of the destination rectangle so that it can center our text on the canvas. Because the x and y values of the dest (destination) rectangle specify the top-left corner of that rectangle, we need to subtract half the width and half the height of the rectangle to make sure that it is centered. The SDL_RenderCopy function then renders this texture to our rendering buffer and SDL_RenderPresent moves that entire buffer to the HTML5 canvas.
At this point, all that is left to do in the code is return:
return EXIT_SUCCESS;
Returning with a value of EXIT_SUCCESS tells our JavaScript glue code that everything went well when running this module.