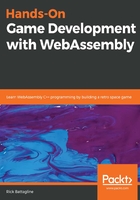
Using the WebAssembly virtual filesystem
The next few lines will open up the TrueType font file in the virtual filesystem, and render it to SDL_Texture, which can be used to render to the canvas:
font = TTF_OpenFont( FONT_FILE, FONT_SIZE );
SDL_Color font_color = {255, 255, 255, 255 }; // WHITE COLOR
SDL_Surface *temp_surface = TTF_RenderText_Blended( font, MESSAGE,
font_color );
texture = SDL_CreateTextureFromSurface( renderer, temp_surface );
SDL_FreeSurface( temp_surface );
In the first line of the preceding code, we open the TrueType font by passing in the location of the file in the WebAssembly virtual filesystem, defined at the top of the program. We also need to specify the font's point size, which was defined as 16 at the top of the program as well. The next thing we do is create an SDL_Color variable that we will use for the font. This is a RGBA color, and we have all values set to 255 so that it is a fully opaque white color. After we have done this, we will need to render the text to a surface using the TTF_RenderText_Blended function. We pass the TrueType font we opened a few lines earlier, the MESSAGE, which was defined as "HELLO SDL!", near the top of the program, and the font color, defined as white. Then, we will create a texture from our surface and free the surface memory we have just allocated. You should always free the memory from your surface pointers immediately after using them to create a texture, as once you have your textures the surfaces are no longer needed.