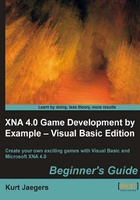
Time for action – creating the GameBoard.cs class
- As you did to create the
GamePiece
class, right-click on Flood Control in Solution Explorer and select Add | Class.... Name the new class fileGameBoard.vb.
- Add the following declarations to the
GameBoard
class:Private rand As New Random() Public Const GameBoardWidth As Integer = 7 Public Const GameBoardHeight As Integer = 9 Private playingPieces As Texture2D Private emptyPiece As Rectangle Private boardSquares(GameBoardWidth, GameBoardHeight) As GamePiece Private waterTracker As New List(Of Vector2)()
What just happened?
We used the Random
class in SquareChase
to generate random numbers. Since we will need to randomly generate pieces to add to the game board, we need an instance of Random
in the GameBoard
class.
The Texture2D
(playingPieces
) and the Rectangle
(emptyPiece
) will be passed in when the class is created and hold the sprite sheet used to draw the board and the location of the sheet's empty piece square.
The two constants and the boardSquares
array provide the storage mechanism for the GamePiece
objects that make up the 8x10
piece board. Note that since arrays start counting at 0
instead of 1
, we use numbers for the width and height that are one lower than the actual number of board squares. This is a personal preference, but we will be doing a lot of looping over the items in the boardSquares
array, and we can either add a -1
to all of the references to our width and height, or declare them as one item smaller to take zero-based arrays into account.
Tip
Visual Basic arrays are a bit quirky in order to maintain some compatibility with pre-.NET versions of VB. In prior versions of VB, arrays could be designated as starting with either element 0
or element 1
. If you declared an array with 5
elements when you had your Option
Base
set to 0
, you actually got 6
elements, numbered 0
through 5
. Visual Basic .NET no longer allows arrays to be one-based, but does continue to allocate the extra array element at the end of the array just like older versions did. For this reason, our code will not actually fail if we had our width and height set to 8
and 10
, but it would produce some strange results. Go ahead and try it out after you have completed the chapter!
Finally, a list of Vector2
objects is declared that we will use to identify scoring pipe combinations. The List
class is one of the .NET Framework's generic collection classes – classes that support the Of
notation that we first saw when loading a texture for SquareChase
. Each of the Collection
classes can be used to store multiple items of the same type, with different methods to access the entries in the collection. We will use several of the Collection
classes in our projects. The List
class is much like an array, except that we can add any number of values at runtime, and remove values in the List
, if necessary.
A Vector2
is a structure defined by the XNA framework that holds two floating point (single) values, X
and Y
. Together, the two values represent a vector pointing in any direction from an imaginary origin (0, 0)
point. We will use Vector2
structures to represent the locations on our game board in Flood Control and off-setting all of our additional drawing based on this location.