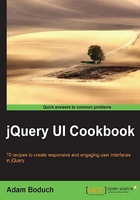
Using effects with the button hover state
The button widget utilizes the various states found in the jQuery UI theme framework. For example, when the user hovers over a button widget, this event triggers a handler inside the button widget that applies the ui-state-hover
class to the element, changing its appearance. Likewise, when the mouse leaves the widget, a different handler removes that class.
This default functionality of the button widget works fine—it just uses the addClass()
and removeClass()
jQuery functions to apply the hover class. As the user moves around and considers what he/she wants to do next, the mouse is likely to move in and out of button widgets; this is where we tweak the experience by providing the user with some subtle effects.
Getting ready
For this example, we'll just create three simple button elements that will serve as the button widgets. This way, we can experiment with moving the mouse pointer over several buttons.
<div> <button>Button 1</button> <button>Button 2</button> <button>Button 3</button> </div>
How to do it...
Let's extend the capabilities of the default button widget to include a new option called animateHover
that when true
, animates the addition and removal of the ui-state-hover
class.
(function( $, undefined ) { $.widget( "ab.button", $.ui.button, { options: { animateHover: false }, _create: function() { this._super( "create" ); if ( !this.options.animateHover ) { return; } this._off( this.element, "mouseenter mouseleave" ); this._on({ mouseenter: "_mouseenter", mouseleave: "_mouseleave" }); }, _mouseenter: function( e ) { this.element.stop( true, true ) .addClass( "ui-state-hover", 200 ); }, _mouseleave: function( e ) { this.element.stop( true, true ) .removeClass( "ui-state-hover", 100 ); } }); })( jQuery ); $(function() { $( "button" ).button( { animateHover: true } ); });
How it works...
We've added a new option to the button widget called animateHover
. When true
, buttons will animate the addition or removal of the CSS properties found in ui-state-hover
class. This is all done by overriding the _create()
method, called when the button widget is first instantiated. Here, we're checking if the animateHover
option is false
, after we call the original _create()
method that performs routine button initialization tasks.
If the option is set, the first job is unbinding the original mouseenter
and mouseleave
event handlers from the button. These handlers are what, by default, simply add or remove the hover class. This is exactly what we want to change, so once the original handlers are removed, we're free to register our own using _on()
. This is where we use the stop()
, addClass()
, and removeClass()
functions. The jQuery UI effects extensions apply to the addClass()
and removeClass()
functions if a duration is given after the class name, which we've done here. We want the adding of the ui-state-hover
class to take 200
milliseconds and the removal of the class to take 100
milliseconds since the initial hover is more noticeable by the user. Finally, the stop( true, true )
call is a common technique in jQuery for ensuring animations don't overlap and cause jittery behavior from the user perspective.