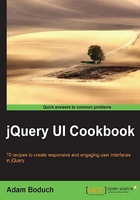
Making simple checklists
Checklists are easy enough to do in plain old HTML, all you really need are some checkboxes and some labels beside them. If you're using a widget framework such as jQuery UI, however, we can enhance that list with ease. The button widget knows how to behave when applied to an input
element of type checkbox
. So let's start off with a basic list and see how we can apply the button widget to the input
elements. We'll also see if we can take the user interactivity a step further with some state and icon enhancements.
Getting ready
Let's start by creating a simple HTML div
to hold our checklist. Inside, each item is represented by an input
element of type checkbox
, along with a label
for the element.
<div> <input type="checkbox" id="first" /> <label for="first">Item 1</label> <input type="checkbox" id="second" /> <label for="second">Item 2</label> <input type="checkbox" id="third" /> <label for="third">Item 3</label> <input type="checkbox" id="fourth" /> <label for="fourth">Item 4</label> </div>
With this markup, we actually have a functioning checklist UI, albeit, a less-than-usable one. We can use the toggling capability of the jQuery UI button widget to encapsulate the label
and the checkbox
together as a checklist item.
How to do it...
We'll introduce the following JavaScript code to collect our checkbox
inputs and use their labels
to assemble the toggle button widget.
$(function() { $( "input" ).button( { icons: { primary: "ui-icon-bullet" } } ); $( "input" ).change( function( e ) { var button = $( this ); if ( button.is( ":checked" ) ) { button.button( "option", { icons: { primary: "ui-icon-check" } }); } else { button.button( "option", { icons: { primary: "ui-icon-bullet" } }); } }); });
With that, you have a toggle-button checklist, complete with icons to assist in conveying the state. When the user clicks on the toggle-button, it goes into "on" state, which is depicted by the change in background color, and other theme properties. We've also added icons that toggle along with the button state.

How it works...
Our event handler, fired when the DOM is ready, requires only one line of code to turn the input
elements on the page into toggle buttons. Within the button constructor, we're specifying that the default icon to use is the ui-icon-bullet
icon class from the theme framework. The button widget knows that we're creating a toggle button because of the underlying HTML element. Since these are checkboxes, the widget will change its behavior when the button is clicked—in the case of a checkbox
, we want the button to give the appearance of toggling on and off. Additionally, the button widget knows which label
belongs to which button based on the for
attribute. For example, the label for for="first"
will be assigned to the button with id="first"
.
Next we apply the change
event handler to all our buttons. This handler is the same for each button, so we can bind it to all buttons at once. The job of this handler is to update the button icon. We don't have to change anything else about the button state because the default button implementation will do that for us. All we need to do in our event handler is check the state on the checkbox
itself. If checked, we show the ui-icon-check
icon. Otherwise, we show the ui-icon-bullet
icon.