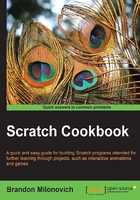
Basic broadcasting and receiving
Broadcasting and receiving is conceptually one of the most challenging ideas we've gotten to so far. That's because the idea is slightly more abstract than some of the other things we've discussed. Let's first talk a little about the principle it is built on, and then go into how it works in Scratch.
Getting ready
We have two separate ideas to think about. The first is the idea of broadcasting. Think of it as a radio station that sends out a signal that only radios can hear. If you are walking around outside, you're not going to hear anything. If you turn on a radio though, you can hear everything being broadcast. Receiving is kind of like the radio. It listens for a specific signal being broadcast, and then will trigger the code placed under it.
In Scratch, we have two blocks that act in this way. The broadcast block and the when I receive block, both of which are Events blocks. We'll talk more about these in the How it works… section coming up.
For this recipe, we will continue using the same Scratch program we have been working with for this chapter. Start off by matching your code with the following two sprites.
Our code for Monkey Mike:

And the code for Frog should be:

Now that you have caught up with us, we're going to have Monkey Mike introduce Frog as the storyteller, and then broadcast to Frog to begin the story.
How to do it...
Follow these steps to program Monkey Mike and Frog:
- Have your Monkey Mike script ready to program.
- At the end of the current script that we have, drag over a say block (with the time element) from the Looks category.
- Type the text Frog will now tell the story. into the block from step 2.
- Return to the Events blocks. We will now drag over the broadcast and wait blocks to the end of the sequence of code.
- Click on the drop-down arrow next to the block you just dragged over and select new message….
- A dialog box will appear. Enter the message name (that you want to broadcast) as Start Story.
Tip
The message name is only for making your code clear to others who look at it or for yourself later. Make it something simple, and something you'll recognize.
- Since we used the version of broadcast that waits for the end of the sequence it starts, we'll need to plan for Monkey Mike to do something when the story is finished. Drag over another say block and add the text That was a good story, Frog. to the block.
- Now that we have finished our broadcast message coming from Monkey Mike, we need to turn our attention to Frog. Be sure you have the script area of Frog selected and look in the Events blocks.
- Drag over the top hat block that says when I receive. Since we already created a broadcast message, you'll see Start Story already filled in for us.
- Now add enough say blocks to tell the story you want to tell. Your overall stack for the when I receive block should look like the following, customized for your own story of course:
- Now, test out your program. You should see what we had before, in addition to Frog telling our "story," with Monkey Mike concluding at the end.
Note
Looking for a better way to view your story? Notice that there is a button just above the stage. This adjusts the interface and brings the stage to full screen. When you want to test your program and don't need to see any code, choose this option to maximize the stage size.
How it works...
First we'll talk about the broadcast and receive blocks.
The broadcast block
The broadcast block has two forms, both of them shown here:

The first of the broadcast blocks simply broadcasts a message. This message is just like when a radio station sends out a signal that only radios can interpret. Thus, the message can only be interpreted in Scratch in a certain way.
The second block sends out a message—just as the first one does—but then waits for everything associated with that message to be completed before continuing the sequence of the program. This will make more sense once you see how this block is used, particularly with the receive block.
The receive block
Our receive block acts somewhat like the radio. It is a top hat block, so triggers a sequence of code just like the green-flag block and other top hat blocks we've worked with so far. This block is a listener. A listener does just what its name suggests; it listens in the background and waits for some event to occur. In this case, that event is some sort of broadcast sent somewhere else in the program. You'll see that whenever you have one of these blocks, either broadcast or receive, you need to have at least one of the other blocks, else nothing will happen.
More about the code
There are two main pieces to this code to understand what is going on in the background. The first of this is what happens with Monkey Mike. Monkey Mike gets the process started by broadcasting the message Start Story. Built into Frog's code we have a listener, the when I receive (Start Story) block. When the listener is triggered by the broadcast from Monkey Mike, the sequence of code connected to the listener (our story) begins to run. The broadcast block we had back in Monkey Mike's code waits until this code is finished. Once it's finished, Monkey Mike continues with what is left to that sequence of code. In our case, all that remains then is to end the story with his final statement.
Tip
Keep in mind that we can have multiple receive blocks working on one message. For instance, the broadcast can be sent and two receive blocks (in different sprites or the same sprites, doesn't matter) pick up the message and begin running a sequence of code.
There's more...
We can use our broadcast
and receive
commands to do any number of things. Let's take a moment to examine two other possibilities here.
Triggering an event with a click
In the previous recipe, we mentioned that you can only use the when this sprite clicked top hat block on the particular sprite being programmed, and not on others. We can use broadcasting and receiving to get around this.
For example, let's assume for a moment that you want to click anywhere on the stage to get Frog to begin moving. Set the visible script area to the stage and drag over the top hat block when this sprite clicked. Underneath this, drag over a new broadcast block (this time, use the one without the wait
command). Click on the drop-down arrow and select New. Give this message the name Move.

Return to the program for Frog and drag over the when I receive block to the script area. Make sure the message you just created, Move, is selected. Now, right-click on the forever loop we created previously for movement and select duplicate (as shown in the following screenshot).

A copy of this sequence of code will appear. Drop it below our new top hat block to have the sequence as shown here:

If you run your program now, you will notice that if you click on the stage as well as Frog, Frog will move faster. This is a result of both scripts running at once, and so the speed is doubled. If you're worried about the speed varying like this, the best suggestion is to not have both sets of code in your program. Since these pieces of code are independent, we cannot (easily) stop one from running.
You can now use this technique to work around clicking on one object and having the code specifically on that object.
Changing the background
What if at some point in your story you want to change the background? Perhaps we can set this to happen when Monkey Mike announces that Frog will tell the story. Recall that we mentioned you can have multiple pieces of receive commands from a single broadcast.
Return to the script area for our stage. Drag over another when I receive block and change the message the block is receiving to Start Story. Next, take a look at your block palette and change the category to Looks. You'll notice that the category appears different for the stage than it does when you are working with a sprite.
Drag over the switch backdrop to () block, the first one in the list, and connect it to the when I receive block that you just dragged over. To make this block effective, you need to select the Backdrops tab and be sure that there is a second background in your program. In our case, we'll select Import and navigate to the bench in a park, as shown in the following screenshot. Then select OK.

The background will automatically change to the new image you just imported, so you'll want to click on the first background to switch back.
Now return to the script area for the stage and adjust the switch backdrop to block to indicate a switch to the new image that we just imported. Now when we run our project, when the story begins, the setting will change to the park.
We should also note that we should drag one more piece of code into the stage area. It is worth noting that we can place this code in any sprite, but it is a common practice to put it in the stage since that is the most relevant area of the program for it.
Drag in a when green flag clicked block as well as the switch to background block. In the block that switches the background, ensure that the original background is selected. By adding this piece of code, you'll be resetting the background to your original image each time you start the story.

Note
Later on when we work with what are called variables, we will call this procedure of resetting things declaring variables. This process ensures that the program starts the same way every time. For instance, in games where we are keeping a tab on the score, we'll want to reset that score to 0 each time someone plays the game.
See also
- See the next recipe, Resetting parts of a program, for a quick description of what other things need to be reset in this story
- We'll explore more graphic effects in the final recipe of this chapter, Other fun graphic effects