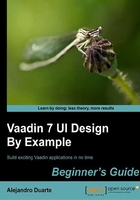
Time for action – adding a combobox
Implement the initCombo
method of your TimeItUI
class as shown in the following code snippet:
private void initCombo() { for(TestSet testSet : testSets) { combo.addItem(testSet); combo.setItemCaption(testSet, testSet.getTitle()); } combo.addValueChangeListener(new ValueChangeListener() { @Override public void valueChange(ValueChangeEvent event) { TestSet testSet = (TestSet) combo.getValue(); textField.setValue("" + testSet.getDefaultTimes()); button.setDescription(testSet.getDescription()); } }); combo.setImmediate(true); }
What just happened?
It's not that complicated. If we isolate the for
portion of the previous code, we'll get this:
for(TestSet testSet : testSets) { combo.addItem(testSet); combo.setItemCaption(testSet, testSet.getTitle()); }
For each TestSet
instance in our array, we add a TestSet
instance and then we say, "Okay, Vaadin for this testSet
array I just added, please show what testSet.getTitle()
returns. Thank you very much". We are adding instances of TestSet
and explicitly specifying what we want to be shown for each option inside the ComboBox
component.
Note
If we don't specify an item caption, Vaadin will call testSet.toString()
and use the returned value as caption. That's the default behavior, but you can use the setItemCaptionMode
method to specify different strategies to get the caption for each item in a ComboBox
instance. If you are curious, take a look at the API documentation for ComboBox
at https://vaadin.com/api/.
Responding to value changes
So, we have our combo
ready and showing all available tests. If you have already played with the application, you may have noted that when you select a test, the number of iterations shown in the text field and the button tooltip explaining the test are updated accordingly. Well, the rest of the initCombo
method implements this functionality.
Do you remember when we added a ClickListener
instance for the button in the previous chapter? We are doing something similar here. This time, we are adding a ValueChangeListener
instance to execute our code when the user selects an option:
combo.addValueChangeListener(new ValueChangeListener() {
@Override
public void valueChange(ValueChangeEvent event) {
// your code here
}
});
Getting and setting the value of input components
The user selects one option, the valueChange
method of our anonymous listener is called, and now we can update the value in textField
. That's one line of code:
textField.setValue(theValue);
Wait... how do we know what is the selected value in the combobox? If setValue
is for setting the value, then getValue
is for getting the value! Unbelievable! Let's get the value:
TestSet testSet = (TestSet) combo.getValue();
Now that we have the value, we can easily set the text in the textField
:
textField.setValue("" + testSet.getDefaultTimes());
We've just learned something valuable (it's not wordplay): input components, they all have the getValue
and setValue
methods.
Tooltips
A tooltip is boxed text that appears when the user holds the mouse pointer over a UI element. The following screenshot shows a Vaadin tooltip:

Adding tooltips is a piece of cake. All we need to do is the following:
button.setDescription(testSet.getDescription());
Most UI components include this method. You can put any HTML you want to nicely format your tooltips.
Immediate mode
There is just one final line that we have not explained yet in the initCombo
method:
combo.setImmediate(true);
This makes the component respond as soon as the user changes its value (and after the component loses focus). If we don't put this line, when the user changes the value, Vaadin could say "Okay, I will wait for some more events before I send that change in combo
". This is not only for the ComboBox
component, all input components have this method too.
Tip
When you are developing Vaadin applications, you may get to a point where you have a listener for a component but the listener is not getting called when expected. Check that you have activated the immediate mode in your component.
Error indicators
We must provide feedback to the user when the input is incorrect. Error indicators are a way to provide such feedback.