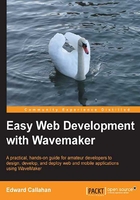
Types
To be more precise about the com.custpurchasdb.data.Lineitem
parameter in our read request, it is actually the type name of the read request. WaveMaker projects define types from primitive types such as Boolean and custom complex types such as Lineitem
. In our runtime read example, com.custpurchasedb.data.Lineitem
is both the package and class name of the imported Hibernate entity and the type name for the line item entity in the project.
Maintaining type information enables WaveMaker to ease a number of development issues. As the client knows the structure of the data it is getting from the server, it knows how to display that data with minimal developer configuration, if any. At design time, Studio uses type information in many areas to help us correctly configure our application. For example, when we set up a grid, type information enables Studio to present us with a list of possible column choices for the grid's dataset type. Likewise, when we add a form to the canvas for a database insert, it is type information that Studio uses to fill the form with appropriate editors.
Line item is a project-wide type as it is defined in the server side. In the process of compiling the project's Java services sources, WaveMaker defines system types for any type returned to the client in a client facing function. To be added to the type system, a class must:
- Be public
- Define public getters and setters
- Be returned by a client exposed function
- Have a service class that extends
JavaServiceSuperClass
or uses the@ExposeToClient
annotation
Tip
WaveMaker 6.5.1 has a bug that prevents types from being generated as expected. Be certain to use 6.5.2 or newer versions to avoid this defect.
It is possible to create new project types by adding a Java service class to the project that only defines types. Following is an example that creates a new simple type called Record
to the project. Our definition of Record
consists of an integer ID and a string. Note that there are two classes here. MyCustomTypes
is the service class containing a method returning the type Record
. As we will not be calling it, the function getNewRecord()
need not do anything other than return a record. Creating a new default instance is an easy way to do this. The class Record
is defined as an inner class. An inner class is a class defined within another class. In our case, Record
is defined within MyCustomTypes
:
// Java Service class MyCustomTypes package com.myco.types; import com.wavemaker.runtime.javaservice.JavaServiceSuperClass; import com.wavemaker.runtime.service.annotations.ExposeToClient; public class MyCustomTypes extends JavaServiceSuperClass { public Record getNewRecord(){ return new Record(); } // Inner class Record public class Record{ private int id; private String name; public int getId(){ return id; } public void setId(int id){ this.id = id; } public String getName(){ return this.name; } public void setName(String name){ this.name = name; } } }
To add the preceding code to our WaveMaker project, we would add a Java service to the project using the class name MyCustomTypes
in the Package and Class Name editor of the New Java Service dialog. The preceding code extends JavaServiceSuperClass
and uses the package com.myco.types
.
A project can also have client-only types using the type definition option from the advanced section of the Studio insert menu. Type definitions are useful when we want to be able to pass structured data around within the client but we will not be sending or receiving that type to the server. For example, we may want to have application scoped wm.Variable
storing a collection of current record selection information. This would enable us to keep track of a number of state items across all pages. Communication with the server is likely to be using only a few of those types at a time, so no such structure exists in the server side. Using wm.Variable
enables us to bind each Record
ID without using code.
The insert type definition menu brings up the Type Definition Generator dialog. The generator takes JSON input and is pre-populated with a sample type. The sample type defines a person object, albeit an unusual one, with a name, an array of numbers for age, a Boolean (hasFoot
), and a related person object, friend
. Replace the sample type with your own JSON structure. Be certain to change the type name to something meaningful. After generating the type, you'll immediately see the newly minted type in type selectors, such as the type field of wm.Variable
.
Tip
Studio is pretty good at recognizing type changes. If for some reason Studio does not recognize a type change, the easiest thing to do is to get Studio to re-read the owning object. If a wm.Variable
fails to show a newly added field to a type in its properties, change the type of the variable from the modified type to some other type and then back again.