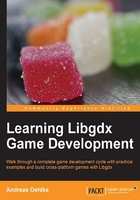
The demo application – how the projects work together
In Chapter 1, Introduction to Libgdx and Project Setup, we successfully created our demo
application, but we did not look at how all the Eclipse projects work together. Take a look at the following diagram to understand and familiarize yourself with the configuration pattern that all of your Libgdx applications will have in common:

What you see here is a compact view of four projects. The demo project to the very left contains the shared code that is referenced (that is, added to the build path) by all the other platform-specific projects. The main class of the demo application is MyDemo.java
. However, looking at it from a more technical view, the main class where an application gets started by the operating system, which will be referred to as Starter Classes from now on. Notice that Libgdx uses the term "Starter Class" to distinguish between these two types of main classes in order to avoid confusion. We will cover everything related to the topic of Starter Classes in a moment.
While taking a closer look at all these directories in the preceding screenshot, you may have spotted that there are two assets
folders: one in the demo-desktop project and another one in demo-android. This brings us to the question, where should you put all the application's assets? The demo-android project plays a special role in this case. In the preceding screenshot, you see a subfolder called data, which contains an image named libgdx.png
, and it also appears in the demo-desktop project in the same place.
Note
Just remember to always put all of your assets into the assets
folder under the demo-android project. The reason behind this is that the Android build process requires direct access to the application's assets
folder. During its build process, a Java source file, R.java
, will automatically be generated under the gen
folder. It contains special information for Android about the available assets. It would be the usual way to access assets through Java code if you were explicitly writing an Android application. However, in Libgdx, you will want to stay platform-independent as much as possible and access any resource such as assets only through methods provided by Libgdx. You will learn more about accessing resources in the last section of this chapter.
You may wonder how the other platform-specific projects will be able to access the very same assets without having to maintain several copies per project. Needless to say that this would require you to keep all copies manually synchronized each time the assets change.
Luckily, this problem has already been taken care of by the generator as follows:
The demo-desktop project uses a linked resource, a feature by Eclipse, to add existing files or folders to other places in a workspace. You can check this out by right-clicking on the demo-desktop project then navigating to Properties | Resource | Linked Resources and clicking on the Linked Resources tab.
The demo-html project requires another approach since Google Web Toolkit (GWT) has a different build process compared to the other projects. There is a special file GwtDefinition.gwt.xml
that allows you to set the asset path by setting the configuration property gdx.assetpath
, to the assets
folder of the Android project. Notice that it is good practice to use relative paths such as ../demo-android/assets
so that the reference does not get broken in case the workspace is moved from its original location. Take this advice as a precaution to protect you and maybe your fellow developers too from wasting precious time on something that can be easily avoided by using the right setup right from the beginning.
The following is the code listing for GwtDefinition.gwt.xml
from demo-html:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE module PUBLIC "-//Google Inc.//DTD Google Web Toolkit trunk//EN" "http://google-web-toolkit.googlecode.com/svn/trunk/ distro-source/core/src/gwt-module.dtd"> <module> <inherits name='com.badlogic.gdx.backends.gdx_backends_gwt' /> <inherits name='MyDemo' /> <entry-point class='com.packtpub.libgdx.demo.client.GwtLauncher' /> <set-configuration-property name="gdx.assetpath" value="../demo-android/assets" /> </module>