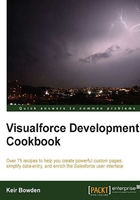
Notifying the containing page controller
In the earlier recipes, we have seen how components can accept an attribute that is a property from the containing page controller and update the value of the property in response to a user action. If the containing page controller needs to determine if the property has changed, it must capture the previous value of the property and compare that with the current value. The same applies if the attribute passed to the component is a field from an sObject managed by the parent page controller.
In this recipe we will create a custom component that can notify its containing page controller when an attribute value is changed. In order to avoid tying the component to a particular page controller class, we will create an interface that defines the method to be used to notify the page controller. This will allow the component controller to notify any page controller that implements the interface.
Note
Interfaces define a "contract" between the calling code and the implementing code. The calling code is able to rely on the method(s) defined in the interface being available without having to know the details of the underlying code that is implementing the interface. This allows the implementing code to be swapped in and out without affecting the calling code.
Getting ready
This recipe requires that you have already completed the Multiselecting related objects recipe, as it relies on the custom sObjects created in that recipe.
How to do it…
- First, create the interface by navigating to the Apex Classes setup page by clicking on Your Name | Setup | Develop | Apex Classes.
- Click on the New button.
- Paste the contents of the
Notifiable.cls
Apex class from the code download into the Apex Class area. - Click on the Save button.
- Next, create the component controller by navigating to the Apex Classes setup page by clicking on Your Name | Setup | Develop | Apex Classes.
- Click on the New button.
- Paste the contents of the
NotifyingMultiSelectRelatedController.cls
Apex class from the code download into the Apex Class area. - Click on the Save button.
- Next, create the custom component by navigating to the Visualforce Components setup page by clicking on Your Name | Setup | Develop | Components.
- Click on the New button.
- Enter
NotifyingMultiSelectRelated
in the Label field. - Accept the default NotifyingMultiSelectRelated that is automatically generated for the Name field.
- Paste the contents of the
NotifyingMultiSelectRelated.component
file from the code download into the Visualforce Markup area and click on the Save button. - Next, create the custom controller for the Visualforce page by navigating to the Apex Classes setup page by clicking on Your Name | Setup | Develop | Apex Classes.
- Click on the New button.
- Paste the contents of the
NotifiableAccountGroupController.cls
Apex class from the code download into the Apex Class area. - Click on the Save button.
- Next, create the Visualforce page by navigating to the Visualforce setup page by clicking on Your Name | Setup | Develop | Pages.
- Click on the New button.
- Enter
NotifiableAccountGroup
in the Label field. - Accept the default NotifiableAccountGroup that is automatically generated for the Name field.
- Paste the contents of the
NotifiableAccountGroup.page
file from the code download into the Visualforce Markup area and click on the Save button. - Navigate to the Visualforce setup page by clicking on Your Name | Setup | Develop | Pages.
- Locate the entry for the NotifiableAccountGroup page and click on the Security link.
- On the resulting page, select which profiles should have access and click on the Save button.
How it works…
Opening the following URL in your browser displays the NotifiableAccountGroup page: https://<instance>/apex/NotifiableAccountGroup
.
Here, <instance>
is the Salesforce instance specific to your organization, for example, na6.salesforce.com, and <account_id>
is the ID of an account from your Salesforce instance.
When accounts are moved between the Available and Selected lists, the component controller notifies the parent page controller, which writes a message into the page with the notification details.

The parent page controller, NotifiableAccountGroupController
, implements the Notifiable
interface.
public with sharing class NotifiableAccountGroupController implements Notifiable
The implementation of the notify
method wraps the notification text in an ApexPages.Message
class and adds this to the messages for the page.
public void notify(String detail) { ApexPages.addMessage(new ApexPages.Message( ApexPages.Severity.INFO, 'Notified - ' + detail)); }
The custom component takes a parameter of type Notifiable
, which is assigned to a property in its controller.
<apex:attribute name="notify" description="The entity to notify when the selection changes" type="Notifiable" assignTo="{!notifiable}" />
The custom component also takes a rerender
parameter, which is used to update part of the containing page when the selection changes.
<apex:attribute name="rerender" description="The component to rerender when the selection changes" type="String" />
In this case, the page passes through the ID of its <apex:pageMessages />
component to display the message added in the page controller's notify
method as described earlier.
When the action methods to move accounts between the Available and Selected lists are executed, these invoke the notify
method with details of the change.
if (null!=notifiable) { notifiable.notify('Values deleted - now ' + selected.value); }
See also
- The Updating attributes in component controllers recipe in this chapter shows how a custom component can update an attribute that is a property of the enclosing page controller.
- The Passing attributes to components recipe in this chapter shows how an sObject may be passed as an attribute to a custom component.
- The Multiselecting related objects in this chapter shows how to create a custom multiselect picklist style component.