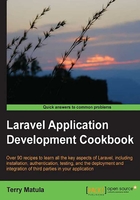
Creating a file uploader
There may be times when we'd like the user to upload a file to our server. This recipe shows how Laravel can handle file uploads through a web form.
Getting ready
To create a file uploader, we need a standard version of Laravel installed.
How to do it...
To complete this recipe, follow these steps:
- Create a route in our
routes.php
file to hold the form:Route::get('fileform', function() { return View::make('fileform'); });
- Create the
fileform.php
View in ourapp/views
directory:<h1>File Upload</h1> <?= Form::open(array('files' => TRUE)) ?> <?= Form::label('myfile', 'My File') ?> <br> <?= Form::file('myfile') ?> <br> <?= Form::submit('Send it!') ?> <?= Form::close() ?>
- Create a route to upload and save the file:
Route::post('fileform', function() { $file = Input::file('myfile'); $ext = $file->guessExtension(); if ($file->move('files', 'newfilename.' . $ext)) { return 'Success'; } else { return 'Error'; } });
How it works...
In our View, we use Form::open ()
and pass in an array with 'files' => TRUE
that automatically sets the enctype in the Form
tag; then we add a form field to take the file. Without using any other parameters in Form::open()
, the form will use the default method of POST
and action of the current URL. Form::file()
is our input field to accept the files.
Since our form is posting to the same URL, we need to create a route to accept the POST
input. The $file
variable will hold all the file information.
Next, we want to save the file with a different name but first we need to get the extension of the uploaded file. So we use the guessExtension()
method, and store that in a variable. Most of the methods for using files are found in Symfony's File libraries.
Finally, we move the file to its permanent location using the file's move()
method, with the first parameter being the directory where we will save the file; the second is the new name of the file.
If everything uploads correctly, we show 'Success'
, and if not we show 'Error'
.
See also
- The Validating a file upload recipe