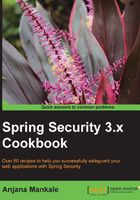
Using Struts 2 with digest/hashing-based Spring Security
Using the form-based or basic authentication doesn't make the Struts 2-based application secure since the passwords are exposed to the user as plain text. There is a crypto package available in Spring Security JAR. The package can decrypt the encrypted password, but we need to tell the Spring Security API about the algorithm used for encryption.
Getting ready
- Create a dynamic web project in Eclipse
- Add the Struts 2 JARs
- Add Spring Security related JARs
- The
web.xml
,struts2.xml
, and JSP settings remain the same as the previous application
How to do it...
Let's encrypt the password: packt123456
.
We need to use an external JAR, JACKSUM
, which means Java checksum. It supports both MD5 and SHA1 encryption.
Download the jacksum.zip
file (http://www.jonelo.de/java/jacksum/#Download) and extract the ZIP folder.
packt>java -jar jacksum.jar -a sha -q"txt:packt123456"

Update the applicationcontext-security.xml
file:
<beans:beans xmlns="http://www.springframework.org/schema/security" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/security http://www.springframework.org/schema/security/spring-security-3.1.xsd"> <global-method-security pre-post-annotations="enabled"> <!-- AspectJ pointcut expression that locates our "post" method and applies security that way <protect-pointcut expression="execution(* bigbank.*Service.post*(..))" access="ROLE_TELLER"/> --> </global-method-security> <http> <intercept-url pattern="/welcome" access="ROLE_TELLER" /> <http-basic /> </http> <authentication-manager> <authentication-provider> <password-encoder hash="sha" /> <user-service> <user name="anjana" password="bde892ed4e131546a2f9997cc94d31e2c8f18b2a" authorities="ROLE_TELLER" /> </user-service> </authentication-provider> </authentication-manager> </beans:beans>
How it works...
We need to update the Applicationcontext-security.xml
file. Observe that the type of authentication is basic but the password is hashed using the algorithm. We want the Spring Security to decrypt it using the SHA algorithm and authenticate the user.
Spring Security is very flexible in handling digest authentication. You can also see that there is no container-based dependency.
Basic authentication from the browser can be seen in the following screenshot:

Spring has authenticated the user by decrypting the password:

See also
- The Displaying custom error messages in Struts 2 for authentication failure recipe
- The Authenticating databases with Struts 2 and Spring Security recipe
- The Authenticating with ApacheDS with Spring Security and Struts 2 application recipe
- The Using Spring Security logout with Struts 2 recipe
- The Getting the logged-in user info in Struts 2 with Spring Security recipe