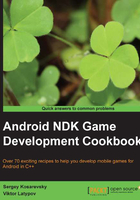
Compiling the native static libraries for Android
Android NDK includes a number of GCC and Clang toolchains for each kind of the supported processors.
Getting ready
When building a static library from the source code, we follow the steps similar to the Windows version.
How to do it...
- Create a folder named
jni
and create theApplication.mk
file with the appropriate compiler switches, and set the name of the library accordingly. For example, one for the FreeImage library should look like the following:APP_OPTIM := release APP_PLATFORM := android-8 APP_STL := gnustl_static APP_CPPFLAGS += -frtti APP_CPPFLAGS += -fexceptions APP_CPPFLAGS += -DANDROID APP_ABI := armeabi-v7a x86 APP_MODULES := FreeImage
- The
Android.mk
file is similar to the ones we have written for the sample applications in the previous chapter, yet with a few exceptions. At the top of the file, some required variables must be defined. Let us see what theAndroid.mk
file for the FreeImage library may look like:# Android API level TARGET_PLATFORM := android-8 # local directory LOCAL_PATH := $(call my-dir) # the command to reset the compiler flags to the empty state include $(CLEAR_VARS) # use the complete ARM instruction set LOCAL_ARM_MODE := arm # define the library name and the name of the .a file LOCAL_MODULE := FreeImage # add the include directories LOCAL_C_INCLUDES += src \ # add the list of source files LOCAL_SRC_FILES += <ListOfSourceFiles>
- Define some common compiler options: treat all warnings as errors (
-Werror
), theANDROID
pre-processing symbol is defined, and thesystem
include directory is set:COMMON_CFLAGS := -Werror -DANDROID -isystem $(SYSROOT)/usr/include/
- The compilation flags are fixed, according to the selected CPU architecture:
ifeq ($(TARGET_ARCH),x86) LOCAL_CFLAGS := $(COMMON_CFLAGS) else LOCAL_CFLAGS := -mfpu=vfp -mfloat-abi=softfp -fno-short-enums $(COMMON_CFLAGS) endif
- Since we are building a static library, we need the following line at the end of the makefile:
include $(BUILD_STATIC_LIBRARY)
How it works...
The Android NDK developers provide their own set of rules to build applications and libraries. In the previous chapter we saw how to build a shared object file with the .so
extension. Here we just replace the BUILD_SHARED_LIBRARY
symbol to the BUILD_STATIC_LIBRARY
and explicitly list the source files required to build each object file.
Note
Of course, you can build a shared library and link your application dynamically against it. However, this usually is a good choice when the library is located in the system and is shared between several applications. In our case, since our application is the sole user of the library, the static linking will make it easier to link and debug the project.