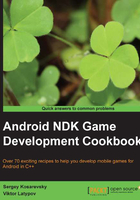
Adding native C++ code to your application
Let us expand our minimalistic Java template, which was discussed in the previous recipe, so we can create a placeholder for our native C++ code.
Getting ready
We need to copy all the files from our App1
project to save time while creating the initial project files. This recipe will focus on the changes to be made to the App1
project in order to add the C++ code to it.
How to do it...
Carry out the following steps to create a placeholder for our C++ code:
- Add the
jni/Wrappers.cpp
file with the following code:#include <stdlib.h> #include <jni.h> #include <android/log.h> #define LOGI(...) ((void)__android_log_print(ANDROID_LOG_INFO, "App2", __VA_ARGS__)) extern "C" { JNIEXPORT void JNICALL Java_com_packtpub_ndkcookbook_app2_App2Activity_onCreateNative( JNIEnv* env, jobject obj ) { LOGI( "Hello World!" ); } }
- We need to change our
Activity
class from the previous recipe to make use of the native code we just added in the preceding section, through the following code:package com.packtpub.ndkcookbook.app2; import android.app.Activity; import android.os.Bundle; public class App2Activity extends Activity { static {
Here we load the native library named
libApp2.so
. Note the omittedlib
prefix and.so
extension:System.loadLibrary( "App2" ); } @Override protected void onCreate( Bundle icicle ) { super.onCreate( icicle ); onCreateNative(); } public static native void onCreateNative(); };
- Tell the NDK build system how to treat the
.cpp
file. Create thejni/Android.mk
file. TheAndroid.mk
file is used by the Android NDK build system to find out how to treat the source code of your project:TARGET_PLATFORM := android-7 LOCAL_PATH := $(call my-dir) include $(CLEAR_VARS) LOCAL_ARM_MODE := arm LOCAL_MODULE := App2 LOCAL_SRC_FILES += Wrappers.cpp LOCAL_ARM_MODE := arm COMMON_CFLAGS := -Werror -DANDROID -DDISABLE_IMPORTGL \ -isystem $(SYSROOT)/usr/include/ ifeq ($(TARGET_ARCH),x86) LOCAL_CFLAGS := $(COMMON_CFLAGS) else LOCAL_CFLAGS := -mfpu=vfp -mfloat-abi=softfp \ -fno-short-enums $(COMMON_CFLAGS) endif LOCAL_LDLIBS := -llog -lGLESv2 -Wl,-s LOCAL_CPPFLAGS += -std=gnu++0x include $(BUILD_SHARED_LIBRARY)
Note the
ifeq ($(TARGET_ARCH),x86)
section. Here we specify architecture-specific compiler flags for floating point support on ARMv7. This will give you hardware floating-point support on the ARM architecture and a warnings-free log on the x86 Android target architecture.. - Paste the following code into the
jni/Application.mk
file:APP_OPTIM := release APP_PLATFORM := android-7 APP_STL := gnustl_static APP_CPPFLAGS += -frtti APP_CPPFLAGS += -fexceptions APP_CPPFLAGS += -DANDROID APP_ABI := armeabi-v7a APP_MODULES := App2 NDK_TOOLCHAIN_VERSION := clang
How it works...
- First of all, we need to compile the native code. From the root of your
App2
project, run the following command:>ndk-build
- You should see the following output:
Compile++ arm: App2 <= Wrappers.cpp SharedLibrary: libApp2.so Install : libApp2.so => libs/armeabi-v7a/libApp2.so
- Now proceed to the
.apk
creation as in the previous recipe by running the following command:>ant debug
- Your
libApp2.so
native shared library will be packed into theApp2-debug.apk
package. Install and run it. It will output aHello World!
string into the device log.
There's more...
You can use the adb
command to view the device log. A nice clean formatted log with timestamps can be created using the following command:
>adb logcat -v time > 1.txt
The actual output from your device will look similar to the following command:
05-22 13:00:13.861 I/App2 ( 2310): Hello World!