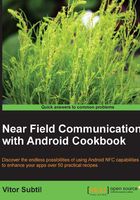
Verifying whether the device has an NFC adapter
The very first lines of code we write in an app that uses NFC should be a simple validation that tests for the NFC adapter.
How to do it…
We'll create an application that shows a Toast saying whether the device has the NFC adapter, as follows:
- Open the previously created
NfcBookCh1Example1
project. - Open
MainActivity
located undernfcbook.ch1.example1
and place the following code inside theonCreate
method:NfcAdapter nfcAdapter = NfcAdapter.getDefaultAdapter(this); if (nfcAdapter != null && nfcAdapter.isEnabled()) { Toast.makeText(this, "NFC is available.", Toast.LENGTH_LONG).show(); } else { Toast.makeText(this, "NFC is not available on this device. This application may not work correctly.", Toast.LENGTH_LONG).show(); }
- Run the application and try enabling and disabling NFC and see the different results.
How it works…
Android provides a simple way to request the Adapter object by calling getDefaultAdapter([context])
. If a valid NfcAdapter
instance is returned, the NFC adapter is available; however, we still need to check if it is enabled by calling the isEnabled()
method. Otherwise, we need to inform the user that the application may not function correctly as NFC is a required feature. Testing the result for a null value is the simplest way to know if NFC is available to us. However, we can also use the hasSystemFeature
method from the PackageManager
class to do this validation.
There's more…
Testing for NFC is an operation that we will probably do very often. So, we can create a simple method and call it every time we need to test for NFC, as shown in the following code:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); if (hasNfc()) { Toast.makeText(this, "NFC is available.", Toast.LENGTH_LONG).show(); } else { Toast.makeText(this, "NFC is not available on this device. This application may not work correctly.", Toast.LENGTH_LONG).show(); } } boolean hasNfc() { boolean hasFeature = getPackageManager().hasSystemFeature(PackageManager.FEATURE_NFC); boolean isEnabled = NfcAdapter.getDefaultAdapter(this).isEnabled(); return hasFeature && isEnabled; }