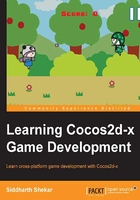
Character movement
For the character to move at a constant speed over a period of time, we need to basically update the position of the character on the x or y axes, depending on the requirement of the game.
So, to achieve this, we use the scheduleUpdate()
function, which is inbuilt in Cocos2d-x. This function will automatically call the update function over and over again, depending on the frames per second (fps) that we set for the applicationDidFinishLaunching()
function of the AppDelegate
class. If you remember, we the set the frame rate to be 1/60
, which is 60 frames per second, so that the update function will also be called 60 times per second.
To include the update function, we go to the init()
function and add the following line of code:
this->scheduleUpdate();
This will initialize a regular call to the update function as soon as the scene is initialized. Next, we need an update function.
Creating a function in Cocos2d-x is similar to creating a function in C++. In the .h
file of HelloWorldScene
, we declare a function as follows:
virtual void update(float dt);
Here, we override the virtual update function of the CCSprite super class. The virtual
keyword is not necessary here; it is just to remind us that this is a virtual function, that's all. The dt
part in float dt
tells the function after how many milliseconds the update function is called again; in this case, it is 1/60, which is approximately 0.0167 seconds.
In the HelloWorldScene.cpp
file, we define the function as follows:
void HelloWorld::update(float dt) { }
Now, to check whether the update function is getting called again and again, we perform a small test by logging something to the output window.
To do so, we right-click on the wp8component
project in the Solution Explorer pane and go to C/C++ | Preprocessor; then, under Preprocessor Definitions, we click on the arrow mark that is facing downward and add the following at the end of the code snippet:
COCOS2D_DEBUG=1
This will enable us to log to the output window. Now in the update(float dt)
function that we just created, we add the following line of code:
CCLog("updating");
Now, we build and run the project by clicking on the green arrow button at the top. After running the project, we should see the desired output in the console:

We can stop the player from running by clicking on the orange stop button at the top of the window. Now, we can start making the character move.
We first make the character move from the left to the right of the screen. To do this, we add the following lines of code in the update(float dt)
function:
CCPoint p = hero->getPosition(); hero->setPosition(ccp(p.x + 5, p.y));
We now build and run the project; we will see the hero move in the right direction.
So, what we are doing here is taking the current position of the hero in every frame and adding 5
to the X
value, and then, setting the position of the hero to this new value. This makes the character move by 5 pixels per frame, which gives movement to the character:

We can see that the hero keeps on going and going, and then goes completely out of the screen.
So, let's add a condition such that if the hero goes off screen, we reset her to the left of the screen.
Let's add the following code snippet in our update function below the move function code we wrote previously:
if((hero->getPositionX() - hero->getContentSize().width/2)> visibleSize.width) { hero->setPosition(ccp(0.0 - hero->getContentSize().width/2, hero->getPositionY())); }
We should see the hero looping around on the screen now. Here, we check whether the player has gone completely out of the right of the screen by adding half of the width of the hero to the current position and resetting her to the left of the screen if the condition is true.
The aforementioned if
condition can be written as: if the current x position of the hero is greater than the width of the screen plus half of the width of the hero, set him to the back of the screen. We can alternatively check whether the hero's x position is equal to the width of the screen and then reset her, but then it wouldn't look very smooth. This is because the hero is reset to the back even when she is not completely off the screen to the right. So, we add the extra half of the width of the character to check whether she has gone completely off the screen.
Now that we have understood how to move the character, let's take a look at some other ways in which the player can be moved.
Let's first take a look at the touch function.