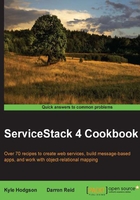
Hosting services from different assemblies
The output of a .NET project is typically an assembly. This applies to ServiceStack projects also. These assemblies can be in the form of a DLL, which could be used by other programs or by a program itself. In this example, we'll show how you can create and reuse assemblies.
In this example, we'll take the ReidsonMessenger
service we've been creating, refactor it a bit to be more modular, and then create a new project to host it.
Getting ready
When we last left off with the ReidsonMessenger service, it had the following structure:

We'll refactor the solution by moving MessengerService
out to its own project, which will leave only the hosting details, AppHost
and Global.asax
, in the main project. Then, we'll create an entirely new solution that hosts the messenger in a console project instead of IIS and add the MessengerService.ServiceModel
and MessengerService.ServiceInterface
DLLs to it as references.
How to do it…
Right-click on the solution and choose Add New Project.
Choose Class Library and name it ReidsonMessenger.ServiceInterface
.
Move ReidsonMessenger
in to this project, and resolve the resulting reference issues by installing ServiceStack and adding a reference to ReidsonMessenger.ServiceModel
:
- Tests will need references to ServiceStack,
ServiceInterface
, andServiceModel
ServiceModel
needs a reference to ServiceStackServiceInterface
needs references to ServiceStack andServiceModel
ServiceClient
needs references to ServiceStack andServiceModel
ReidsonMessenger
needs references to ServiceStack,ServiceInterface
, andServiceModel
Rebuild the solution and make sure your tests pass—we now have a fairly well-structured solution with components that could be hosted in a variety of different ways.
The new structure should look like this:

Now that we have cleaned up our project structure, let's create a new hosting project. We'll call it ConsoleHostedMessneger
. Choose the Console Application project type.
Add an AppHost
class that extends AppSelfHostBase
instead of AppHostBase
, as follows:
class ConsoleAppHost : AppSelfHostBase { public ConsoleAppHost() : base("ReidsonIndustries Console Messaging Service", typeof (MessengerService).Assembly) { } public override void Configure(Funq.Containercontainer) { } }
Now that we have an AppHost
class suitable for self-hosting, we can change our program class' Main
method to initialize the service, as follows:
public class Program { static void Main(string[] args) { var listeningOn = "http://localhost:2202/"; new ConsoleAppHost() .Init() .Start(listeningOn); Console.WriteLine("Listening on {0}", listeningOn); Console.WriteLine("Push any key to stop."); Console.ReadKey(); } }
The resulting service should look like this:

How it works…
Adding the assembly reference to the ServiceInterface
project gives our new solution access to the MessengerService
service from the other project just by referencing the DLL. Adding the assembly reference to the ServiceModel
project gives our new solution access to the different types required to use the ServiceModel project
. We can then easily host these services in an entirely new way by creating a custom AppHost
.
ServiceStack's AppSelfHostBase
gives us access to HttpListener
and a high-performance self-hosting option that doesn't require IIS. We could just as easily have used this technique to add ReidsonMessager
to an entirely different solution to add the services to the new solution.
This provides a flexible way to reuse services in other solutions.
There's more…
There is an important difference between different hosts regarding threading. The ASP.NET host, AppHostBase
, leaves the thread creation up to the web server. ServiceStack does not create any new threads. The AppHostHttpListenerBase class
, which is used by AppSelfHostBase
, creates a single new thread on startup. These different concurrency models should be taken into consideration when deciding which host to use for different tasks.