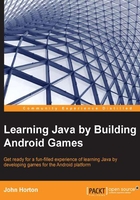
Using the Android Studio visual designer
The Android Studio editor window is a very dynamic area. It presents different file types in the most useful way possible. A little earlier, when we created our project, it also made a basic UI for us. UIs in Android can be built-in Java code or, as we will see, in a visual designer without the need for a single line of Java. However, as we will investigate after we have built the UI of our game menu, to get the UI to do anything useful, we need to interact with it. This interaction is always done with Java code. The visual designer also generates the UI code for us. We will take a very quick look at that too.
As the book progresses, we will mainly shy away from Android UI development, as that is a staple of more non-game apps. We will instead spend more time looking at directly drawing pixels and images to make our games. Nonetheless, the regular Android UI has its uses, and the Android Studio visual designer is the quickest way to get started.
Let's have a look at that now:
- In the Android Studio Project Explorer, double-click on the
layout
folder to reveal theactivity_main.xml
file within it. This should be easy to see unless you have collapsed the directories. If you can't see thelayout
folder, navigate to it using the Project Explorer. It can be found atMath Game Chapter2/src/main/res/layout
via the Android Studio Project Explorer, as shown in the following screenshot: - Now double-click on activity_main.xml to open it in the editor window. After a brief loading time, you will see something very similar to the next screenshot. The following screenshot shows the entire contents of what previously contained just our code. As you can see, what was just a text window now has multiple parts. Let's take a closer look at this screenshot:
In the preceding screenshot labeled (1), called Palette, you can choose from the available Android UI elements and simply click and drag them onto your UI design. Area (2) is the visual view of the UI you are building, where you will click and drag elements from the palette. To the right of the visual UI view, you will see the Component Tree area (3). The component tree allows you to examine the structure of the complex UI and select specific elements more easily. Under this tree is the Properties panel (4). Here you can adjust the properties of the currently selected UI element. These can be simple things such as color and size or much more advanced properties.
Note
Note the tabs labelled (5). These tabs allow you to switch between the two main views that Android Studio provides for this type of layout file. These views, as you can see, are Design and Text. The design view is the default view and is shown in the previous screenshot. The text view also shows your under-construction UI, but it shows the code that has been autogenerated for us instead of the Palette element and the component tree.
We don't need to worry about this code as it is all handled for us. It can be good to look on this tab from time to time so that we can begin to understand what the design tool generates for us. But it is not necessary to do this to learn Java. This code is called eXtensible Markup Language (XML).
- Take a quick look at the Text tab, click on the Design tab when you're done, and we will move on.
Now we have seen an overview of the visual designer and an even briefer glimpse of the automatically generated code that it generates for us. We can take a closer look at some of the actual UI elements that we will be using in our project.
Android UI types
We will now take a whirlwind tour of some really useful Android UI elements, a few key properties, and how to add them together to make a UI. These will introduce us to some of the possibilities as well as how to use them. We will then quickly use what we know to make our menu.
On the visual UI area, click on the words Hello world!. What we have just selected is a widget known as a TextView. TextViews can be small text like this one or large heading type text, which might be useful in our game menu.
Let's try dragging and dropping another TextView onto our visual UI:
- Directly under the Widgets heading in our palette, you can see there are multiple types of TextView. They are presented in the palette as Plain TextView, Large Text, Medium Text and Small Text. Drag and drop a Large Text widget onto our visual design. Don't let go straightaway. As you drag it around the image of the phone, notice how Android Studio graphically shows you different positioning options. In the following screenshot, you can see what the designer looks like when the widget being dragged is positioned at the center:
- Let go of the left mouse button where you want the widget to go. If you let go when it is positioned as shown in the previous screenshot, then the text will appear in the center as expected.
- Now we can play with the properties. In the Properties window, click just to the right of textSize. You might need to scroll to find it. Type
100sp
as the value and press the Enter key. Notice that the text gets much larger. We can refine the size of our text by increasing and decreasing the value entered here. The unitsp
stands for scaled pixels, and is simply a measuring system that attempts to scale the text to an appropriate equivalent actual size across different screen densities. - Play with some more properties if you like and when you're done, click on the TextView we just created in the visual designer to highlight it. Then tap on the Delete key to get rid of it. Now delete the TextView that was present when we started—the one that says Hello world!.
Now you have an apparently empty screen. However, if you click anywhere on the design preview, you will see that we still have some options in the Properties window. This element is called a RelativeLayout. It is one of several layout element types provided as a base to control and align the layout widgets such as buttons, text, and so on. If you look at the top of the Palette window, you will see the main layout options. We will use this layout element when we actually build our game menu in a moment.
ImageViews unsurprisingly are for displaying images. In the standard Android UI, this is a really quick way to add our designers' artwork to our game:
- Drag and drop an ImageView element onto the design in the same way as you positioned the TextView a moment ago. The ImageView element can be found below the Widgets heading. Now position it centrally as before or play with the options by dragging it around the design. We will delete it in a minute; we are just having a bit of an exploration before we do this for real.
- In the Properties window, select the src property in the same way as you selected the textSize property previously.
- Notice that after you select it, you have the option to click on ... to give you more options. Click on ... and scroll to the bottom of the list of options. These are all the image files that we can display in this ImageView. Just for fun, scroll to the bottom of the list, choose ic_launcher, and click on OK. We can make any image we like available and this is a simple, powerful way to build an attractive game menu screen.
- Change the layout:width property to
150dp
and the layout:height property to150dp
. The unit dp is a way of sizing elements and widgets that remains relatively constant across devices with screens that have very different numbers of pixels. - Delete the ImageView in exactly the same way as you deleted the other views previously.
The use of ButtonView is probably given away by its name. Try to click and drag a few buttons onto our layout. Notice that there are a few types of ButtonView, such as Small Button, Button, and, if you look further down the Widget list, ImageButton. We will be using the regular ButtonView, labelled simply as Button.
Now we will do something with each of these Android UI elements combined to make our game menu.
All of the code in this book is organized in projects. If a project spans more than one chapter, a project is provided for each chapter in its finished state. This helps you see the progression and not just the end result. All you need to do to open the project in Android Studio is explained as follows:
- Download the code for this book.
- In Android Studio from the menu bar, navigate to File | Close project.
- Now create a new blank project as we did previously. Browse to where you downloaded the code for this book.
- Navigate to the
Chapter2/MathGameChapter2
folder. Here you will find the code for all the files we create in this chapter. - Open the code files using a plain text editor such as the free Notepad++.
- Copy and paste in your Android Studio project or just compare the code as you see it.
Tip
Although every line of code required in this book is supplied for your convenience, you still need to create each project for yourself through Android Studio. You can then simply copy and paste either the code in its entirety in the file with the matching name, or just the part of the code that you might be struggling with. Keep in mind that if you create a project with a different package name, then you must omit the line of code that is the package name from the supplied code files. The reasons for this will be clearer when we talk more about packages later in the chapter.
Let's actually see how to do it all for ourselves.
Making our game menu
For now we will just make our game menu functional. Later in Chapter 5, Gaming and Java Essentials,we will see how we can make it look good by adding some cool animation to make the menu more visually interesting and fun.
Here is what we are aiming for in this tutorial:

Before you start coding, you should design your layouts on paper first. However, the Android Studio designer is so friendly there is a strong argument, especially for simple layouts, to refine your design actually in the layout designer. Perform the following steps to create the game menu:
- Delete all widgets from your designer by clicking on them one at a time and then tapping the Delete key on each in turn. Be careful not to delete the RelativeLayout layout element as we are going to use it as a base for all the other elements.
- Click and drag a Large Text element from the palette to the top center of the design area and give it the following properties. Remember that you can change properties in the Properties panel by clicking to the right of the property to be changed. Change the text property to
My Math Game
and size to30sp
. - Click and drag an ImageView element from the palette to the center of the design, slightly below the previous TextView. Change the layout:width property to
150dp
and the layout:height property to150dp
. - Now click and drag three buttons for Play, High Scores and Quit. Center them vertically, below the previous ImageView and one below the other, as per our design shown previously.
- Click on the top button, configure the text property, and enter the value
Play
. - Click on the middle button, configure the text property, and enter the value
High Scores
. - Click on the lowest button, configure the text property, and enter the value
Quit
. - As the buttons now contain different amounts of text relative to each other, they will be of slightly different sizes. You can even them up to match the intended layout by clicking and dragging the edges of the smaller buttons to match the larger ones. This is done in mostly the same way as you might resize an application window in Windows.
- Save the project with Ctrl + S or by navigating to File | Save All.
Tip
If you are going to be testing your games on a much larger or much smaller screen than the Nexus 4 shown in the designer, then you might like to adjust the values of the
sp
anddp
units used in this tutorial.A full discussion of Android UI on multiple devices is beyond the scope of this book and is not necessary to make any of the games in this book. If you want to start designing for different screens right away, take a look at http://developer.android.com/training/multiscreen/index.html.
You can view what your menu looks like on other devices simply by selecting the device from the drop-down menu shown in the following screenshot:

Before we make our menu come to life on an actual device, let's take a look at the structure of an Android app and how we can use that structure when writing our Java code.