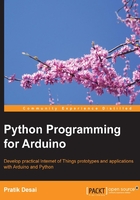
Bridging pySerial and Firmata
In the Firmata section, we already learned how useful it is to use the Firmata protocol instead of constantly modifying the Arduino sketch and uploading it for simple programs. pySerial
is a simple library that provides a bridge between Arduino and Python via a serial port, but it lacks any support for the Firmata protocol. As mentioned earlier, the biggest benefit of Python can be described in one sentence, "There is a library for that." So, there exists a Python library called pyFirmata
that is built on pySerial
to support the Firmata protocol. There are a few other Python libraries that also support Firmata, but we will only be focusing on pyFirmata
in this chapter. We will be extensively using this library for various upcoming projects as well:
- Let's start by installing
pyFirmata
just like any other Python package by using Setuptools:$ sudo pin install pyfirmata
In the previous section, while testing
pySerial
, we uploaded theDigitalSerialRead
sketch to the Arduino board. - To communicate using the Firmata protocol, you need to upload the StandardFirmata sketch again, just as we did in the Uploading a Firmata sketch to the Arduino board section.
- Once you have uploaded this sketch, open the Python interpreter and execute the following script. This script imports the
pyfirmata
library to the interpreter. It also defines the pin number and the port.>>> import pyfirmata >>> pin= 13 >>> port = '/dev/ttyACM0'
- After this, we need to associate the port with the microcontroller board type:
>>> board = pyfirmata.Arduino(port)
While executing the previous script, two LEDs on the Arduino will flicker as the communication link between the Python interpreter and the board gets established. In the Testing the Firmata protocol section, we used a prebuilt program to turn an LED on and off. Once the Arduino board is associated to the Python interpreter, these functions can be performed directly from the prompt.
- You can now start playing with Arduino pins. Turn on the LED by executing the following command:
>>> board.digital[pin].write(1)
- You can turn off the LED by executing the following command. Here, in both commands, we set the state of digital pin 13 by passing values
1
(High) or0
(Low):>>> board.digital[pin].write(0)
- Similarly, you can also read the status of a pin from the prompt:
>>> board.digital[pin].read()
If we combined this script in an executable file with a .py
extension, we can have a Python program that can be run directly to control the LED rather than running these individual scripts on a terminal. Later, this program can be extended to perform complex functions without writing or changing the Arduino sketch.