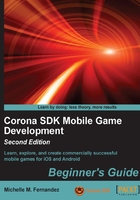
Valuable variables
Like in many scripting languages, Lua has variables. You can think of a variable as something that stores values. When you apply a value to a variable, you can refer to it using the same variable name.
An application consists of comments, blocks, statements, and variables. A comment is never processed, but it is included to explain the purpose of a statement or block. A block is a group of statements. Statements provide instructions on what operations and computations need to be done; variables store the values of these computations. Setting a value in a variable is called assignment.
Lua uses three kinds of variables, which are as follows:
- Global variables
- Local variables
- Table fields (properties)
Variables take up memory space, which can be limited on various mobile devices. When a variable is no longer required, it is best to set its value to nil so that it can be cleaned quickly.
Global variables
A global variable can be accessed in every scope and can be modified from anywhere. The term "scope" is used to describe the area in which a set of variables is accessible. You don't have to declare a global variable. It is created as soon as you assign a value to it:
myVariable = 10 print( myVariable ) -- prints the number 10
Local variables
A local variable is accessed from a local scope and usually called from a function or block of code. When we create a block, we are creating a scope in which variables can live or a list of statements, which are executed sequentially. When referencing a variable, Lua must find the variable. Localizing variables helps speed up the look-up process and improves the performance of your code. Using the local statement, it declares a local variable:
local i = 5 -- local variable
The following lines of code show how to declare a local variable in a block:
x = 10 -- global 'x' variable local i = 1 while i <= 10 do local x = i * 2 -- a local 'x' variable for the while block print( x ) -- 2, 4, 6, 8, 10 ... 20 i = i + 1 end print( x ) -- prints 10 from global x
Table fields (properties)
Table fields are groups of variables uniquely accessed by an index. Arrays can be indexed with numbers and strings or any value pertaining to Lua, except nil
. You index into the array to assign the values to a field using integers or strings. When the index is a string, the field is known as a property. All properties can be accessed using the dot operator (x.y
) or a string (x["y"]
) to index into a table. The result is the same:
x = { y="Monday" } -- create table print( x.y ) -- "Monday" z = "Tuesday" -- assign a new value to property "Tuesday" print( z ) -- "Tuesday" x.z = 20 -- create a new property print( x.z ) -- 20 print( x["z"] ) -- 20
More information relating to tables will be discussed later in the section called Tables.
You may have noticed additional text in certain lines of code in the preceding examples. These are what you call comments. Comments begin with a double hyphen, --
, anywhere except inside a string. They run until the end of the line. Block comments are available as well. A common trick to comment out a block is to surround it with --[[
.
Here is an example of how to comment one line:
a = 2 --print(a) -- 2
This is an example of a block comment:
--[[ k = 50 print(k) -- 50 --]]