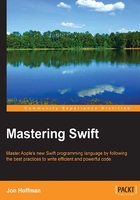
The string type
A string is an ordered collection of characters, such as Hello
or Swift
. In Swift, the string type represents a string. We have seen several examples of strings already in this book, so the following code should look familiar. This code shows how to define two strings:
var stringOne = "Hello" var stringTwo = " World"
Since a string is an ordered collection of characters, we can iterate through each character of a string. The following code shows how to do this:
var stringOne = "Hello" for char in stringOne { println(char) }
The preceding code will display the results shown in the following screenshot:

There are two ways to add one string to another string. We can concatenate them or we can include them inline. To concatenate two strings, we use the +
or the +=
operator. The following code shows how to concatenate two strings. The first example appends stringB
to the end of stringA
and the results are put into a new stringC
variable. The second example appends stringB
directly to the end of variable stringA
without creating a new string:
var stringC = stringA + stringB stringA += stringB
To include a string inline, with another string, we use a special sequence of characters \( )
. The following code shows how to include a string inline with another string:
var stringA = "Jon" var stringB = "Hello \(stringA)"
In the previous example, stringB
will contain the message Hello Jon
because swift will replace the \(stringA)
sequence of characters with the value stringA
.
In Swift, define the mutability of variables and collections by using the var
and let
keywords. If we define a string as a variable using var
, then the string is mutable, which means we can change and edit the value of the string. If we define a string as a constant using let
, then the string is nonmutable, which means we cannot change or edit the value. The following code shows the difference between a mutable string and a nonmutable string;
var x = "Hello" let y = "HI" var z = " World" //This is valid, x is mutable x += z //This is invalid, y is not mutable. y += z
Strings in Swift have three properties that can convert the case of the string. These properties are capitalizedString
, lowercaseString
, and uppercaseString
. The following example demonstrates these properties:
var stringOne = "hElLo" println("capitalizedString: " + stringOne.capitalizedString) println("lowercaseString: " + stringOne.lowercaseString) println("uppercaseString: " + stringOne.uppercaseString)
If we run this code, the results will be as follows;
capitalizedString: Hello lowercaseString: hello uppercaseString: HELLO
Swift provides four ways to compare a string; these are string equality
, prefix equality
, suffix equality
, and isEmpty
. The following example demonstrates these:
var stringOne = "Hello Swift" var stringTwo = "" stringOne.isEmpty //false stringTwo.isEmpty //true stringOne == "hello swift" //false stringOne == "Hello Swift" //true stringOne.hasPrefix("Hello") //true stringOne.hasSuffix("Hello") //false
We can replace all occurrences of a target string with another string. This is done with stringByReplacingOccurrencesOfString
. The following code demonstrates this:
var stringOne = "one,to,three,four" println(stringOne.stringByRelacingOccurrencesOfString("to", withString: "two"))
The preceding example will print one,two,three,four
to the screen because we are replacing all occurrences of to
with two
.
We can also retrieve substrings and inpidual characters from our strings. The following examples show various ways to do this:
var path = "/one/two/three/four" //Create start and end indexes var startIndex = advance(path.startIndex, 4) var endIndex = advance(path.startIndex, 14) path.substringWithRange(Range(start:startIndex, end:endIndex)) //returns the String /two/three path.substringToIndex(startIndex) //returns the String /one path.substringFromIndex(endIndex) //returns the String /four last(path) //returns the last character in the String r first(path) //returns the first character in the String /
In the preceding example, we use the substringWithRange()
function to retrieve the substring between a start and end index. The indexes are created with the advance()
function, as shown in the example. The substringToIndex()
function creates a substring from the beginning of the string to the index. The substringFromIndex()
function creates a substring from the index to the end of the string. We then used the last()
function to get the last character of the string and the first()
function to get the first character.
We can retrieve the number of characters in a string by using the countElements()
function. The following example shows you how to use this function:
var path = "/one/two/three/four" var length = countElements(path)
Finally, Swift makes it very easy to work with paths. These paths can be filesystem paths or full URL paths. The following examples show how to work the path properties of strings in Swift:
var path1 = "/one/two/three/four" path1.pathComponents //returns an array containing "/","one","two","three","four" path1.stringByAppendingPathComponent("five") //returns a new string containing /one/two/three/four/five path1.stringByDeletingLastPathComponent //returns a new string containint /one/two/three path1.lastPathComponent //returns a new string containing four
This completes our whirlwind tour of strings. I know we went through these properties and functions very quickly, but we will be using strings extensively throughout this book, so we will have a lot of time to get used to them.