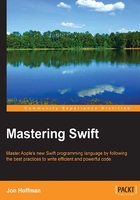
Defining constants and variables
Constants and variables must be defined prior to using them. To define a constant, you use the keyword let
, and to define a variable, you use the keyword var
. The following are some examples of constants and variables:
// Constants let freezingTemperatureOfWaterCelsius = 0 let speedOfLightKmSec = 300000 // Variables var currentTemperature = 22 var currentSpeed = 55
We can declare multiple constants or variables in a single line by separating them with a comma. For example, we could shrink the preceding four lines of code down to two lines like this:
// Constants let freezingTempertureOfWaterCelsius = 0, speedOfLightKmSec = 300000 // Variables var currentTemperture = 22, currentSpeed = 55
We can change the value of a variable to another value of a compatible type; however, as we noted earlier, we cannot change the value of a constant. Let's look at the following Playground. Can you tell what is wrong with the code from the error message that is shown in the following screenshot:

Did you figure out what was wrong with the code? Any physicist can tell you that we cannot change the speed of light, and in our code, the speedOfLightKmSec
variable is a constant, and so we cannot change it either. Therefore, when we try to change the speedOfLIightKmSec
constant, an error is reported by the compiler. We are able to change the value of the highTemperture
variable without an error. We mentioned the difference between variables and constants a couple of times because it is a very important concept to grasp, especially when we define mutable and nonmutable collection types later in Chapter 3, Using Collections and Cocoa Data Types.
Type safety
Swift is a type-safe language. In a type-safe language, we are required to be clear on the types of values we store in a variable. We will get an error if we attempt to assign a value to a variable that is of a wrong type. The following Playground shows what happens if we attempt to put a string value into a variable that expects int values. Note that we will go over the most popular types a little later in the chapter.

Swift performs a type check when it compiles code; therefore, it will flag any mismatched types with an error. The error message in this Playground explains pretty clearly that we are trying to insert a string literal into an int variable.
So the question is, how does Swift know that integerVar
is an int type? Swift uses type inference to figure out the appropriate type. Let's take a look at what type inference is.
Type inference
Type inference allows us to omit the variable type when we define it. The compiler will infer the type based on the initial value. For example, in Objective-C, we would define an integer like this:
int myInt = 1;
This tells the compiler that the myInt
variable is of type int
and the initial value is the number 1
. In Swift, we would define the same integer like this:
var myInt = 1
Swift infers that the variable type is an integer because the initial value is an integer. Let's take a look at a couple more examples:
var x = 3.14 // Double type var y = "Hello" // String type var z = true // Boolean type
In the preceding example, the compiler will correctly infer that variable x
is a Double
, variable y
is a String
, and variable z
is a Boolean
based on the initial values.
Explicit types
Type inference is a very nice feature in Swift and one that you will probably get used to very quickly; however, there are times that we would like to explicitly define a variable's type. For example, in the preceding example, variable x
is inferred to be a Double
, but what if we wanted the variable type to be a Float
? We can explicitly define a variable type like this:
var x : Float = 3.14
Notice the: Float
is after the variable identifier; this tells the compiler to define the variable type to be a float type and gives it an initial value of 3.14
. When we define a variable in the manner, we need to make sure that the initial value is of the same type we are defining the variable to be. If we try to give a variable an initial value that is a different type than we are defining it as, we will receive an error.
We would also explicitly define the variable type if we were not setting an initial value. For example, the following line of code is invalid because the compiler does not know what type to set variable x
to:
var x
If we use this code in our application, we will receive a Type annotation missing in pattern
error. If we are not setting an initial value for a variable, we are required to define the type like this:
var x : Int
Now that we have seen how to explicitly define a variable type, let's take a look at some of the most commonly used types.