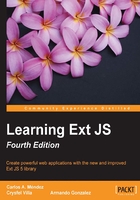
Ajax
Before we start learning about the data package it's important to know how we can make an Ajax request to the server. The Ajax request is one of the most useful ways to get data from the server asynchronously. This means that the JavaScript loop is not blocked while the request is being executed and an event will be fired when the server responds; this allows us to do anything else while the request is being performed.
If you are new to Ajax, I recommend you read more about it. There are thousands of tutorials online, but I suggest you read this simple article at https://developer.mozilla.org/en-US/docs/AJAX/Getting_Started.
Ext JS provides a singleton object (Ext. Ajax
) that is responsible for dealing with all the required processes to perform a request in any browser. There are a few differences in each browser, but Ext JS handles these differences for us and gives us a cross browser solution to make Ajax requests.
Let's make our first Ajax call to our server. First, we will need to create an HTML file and import the Ext library. Then, we can add the following code inside the script
tag:
Ext.Ajax.request({ url:"serverside/myfirstdata.json" }); console.log("Next lines of code...");
Using the request
method, we can make an Ajax call to our server. The request
method receives an object containing the configurations for the Ajax call. The only configuration that we have defined is the URL where we want to make our request.
It's important to note that Ajax is asynchronous by default. This means that once the request
method is executed the JavaScript engine will continue executing the lines of code following it, and it doesn't wait until the server responds. You can also run Ajax in a synchronous way, setting the property Ext.Ajax.async = false
.
Note
For more details, take a look at http://docs.sencha.com/extjs/5.1/5.1.1-apidocs/#!/api/Ext.Ajax-cfg-async.
In the previous code, we did not do anything when the server responded to our request. In order to get the response date, we need to configure a callback
function to execute when the server responds, and also, we have functions for success
or failure
. Let's modify our previous example to set up those callbacks:
Ext.Ajax.request({ url:"serverside/myfirstdata.json", success: function(response,options){ console.log('success function executed, here we can do some stuff !'); }, failure: function(response,options){ Ext.Msg.alert("Message", 'server-side failure with status code ' + response.status); }, callback: function( options, success, response ){ console.log('Callback executed, we can do some stuff !'); } });
The success
function will be executed only when the server responds with a 200-299 status, which means that the request has been made successfully. If the response status is 403, 404, 500, 503, and any other error status, the failure
callback will be executed.
Each function (success or failure) receives two parameters. The first parameter is the server response object, where we can find the response text and headers. The second parameter is the configuration option that we used for this Ajax request, in this case the object will contain three properties: the URL and the success
and failure
callbacks.
The callback
function will be executed always, no matter if it's a failure or success. Also, this function receives three parameters: options
is a parameter to the request call, success
is a Boolean value according to if the request was successful or not, and the response
parameter is an XMLhttpRequest
object that contains the information of the response.
At this point, we have our callbacks set, but we're not doing anything inside yet. Normally, we need to get the data response and do something with it; let's suppose we get the following JSON in our response:
{ "success": true, "msg": "This is a success message..!" }
Tip
In the Ext JS community, one of the preferred formats to send and receive data to the server is JSON; Ext JS can also handle XML. JSON stands for JavaScript Object Notation. If you are not familiar with JSON, you can visit http://www.json.org/ in order to understand more about JSON.
For the success
function to take interaction with the data returned, we need to decode the returned JSON data (which comes in text format), and convert the text to an object so we can access its properties in our code. Let's change the following code in the success
callback:
success: function(response,options){ var data = Ext.decode(response.responseText); Ext.Msg.alert("Message", data.msg); },
First we get the server response as a text using the responseText
property from the response
object. Then, we use the Ext.decode
method to convert the JSON text into JavaScript objects and save the result in a data
variable.
After we have our data object with the server response, we will show an alert message accessing the msg
property from the data object. Let's keep in mind that if we want to show something using the DOM, we need to put our code inside the onReady
method that we have learned in the previous chapter.
Ext.Ajax.request({
url: "serverside/myfirstdata.json ",
success: function(response,options){
console.log('success function executed, here we can do some stuff !');
},
failure: function(response,options){
console.log('server-side failure with status code ' + response.status);
},
callback: function( options, success, response ){
if(success){
var data= Ext.decode(response.responseText);
Ext.Msg.alert("Message", data.msg);
}
}
});
Tip
It's important for the server-side files to return a proper, error-free response; this means that we need to be sure that the server-side files have the proper syntaxes and no warning or error show (PHP as an example).
Also, it's important to specify, the Header on the server side to ensure proper content. For example, header('Content-Type: application/json');
.
If we refresh our browser to execute the code we have modified, we should see something like the following screenshot:
Now, let's assume that we want to use XML instead of JSON. We will create the request in a very similar way to our previous code. The following code should be saved in a new file at serverside/data.xml
:
<?xml version="1.0" encoding="UTF-8"?> <response success="true"> <msg>This is a success message in XML format</msg> </response>
Then, let's proceed to change the URL and the code in the success
callback, as follows:
Ext.Ajax.request({ url: "serverside/myfirstdata.xml", success: function(response,options){ var data = response.responseXML; var node = xml.getElementsByTagName('msg')[0]; Ext.Msg.alert("Message", node.firstChild.data ); }, failure: function(response,options){ Ext.Msg.alert("Message", 'server-side failure with status code ' + response.status); } });
We use the responseXML
property to get the tree of nodes, and then we get the node with a msg
tag. After that, we can get the actual text using the firstChild.data
property from the previous node. If we execute the code, we will see something very similar to our previous example with JSON.
As we can notice, it is easier to work with JSON. We just need to decode the text and then we can use the objects. XML is a little bit complicated, but we can also use this format if we feel comfortable with it.
Passing parameters to Ajax request
It's usually in our applications that we need to pass some parameters to the Ajax request in order to get the proper information. To pass parameters we will use the following code:
Ext.Ajax.request({ url: "serverside/myfirstparams.php", method: 'POST', params: { x:200, y:300 }, success: function(response,options){ var data = Ext.decode(response.responseText); Ext.Msg.alert("Message", data.msg); }, failure: function(response,options){ Ext.Msg.alert("Message", 'server-side failure with status code' + response.status); Ext.Msg.alert("Message", 'server-side failure:' + response.status); } });
Using the params
property, we can set an object of parameters. In this case, we will send only two parameters: x
and y
, but we can send as many as we need. Notice that we set the method
property with the POST
value; by default, Ext JS uses the GET
value for this property, and if we use GET
, the values will be embedded on the URL for request. When we run this code, we'll get the following screenshot:
Tip
Notice that you can return strings in an HTML format (msg
value in this case) to give visual enhancements to the response if you are using Ext.Msg.alert
Setting timeout to Ajax request calls
Sometimes, but not all the time, the server may take too long to respond, so, by default, Ext JS has a configured time of 30 seconds to wait for the response. According to our needs, we can decrease or increase this time by setting the timeout property on the Ajax request configuration. The next example shows us how:
Ext.Ajax.request({
url: "serverside/myfirstparams.php",
method: 'POST',
params: {x:200, y:300},
timeout: 50000,
success: function(response,options){
var data = Ext.decode(response.responseText);
Ext.Msg.alert("Message", data.msg);
},
failure: function(response,options){
Ext.Msg.alert("Message", 'server-side failure with status code ' + response.status);
Ext.Msg.alert("Message", 'server-side failure:' + response.status);
}
});
We have increased the timeout
property to 50 seconds (50000 milliseconds); now our request will be dropped after 50 seconds of waiting for the response.
Tip
You can assign a global timeout value for the whole application setting, changing the value in Ext.Ajax.timeout
(by default it has the value of 30000
). The previous example shows how to set timeouts on independent calls.
If we look into the documentation, we will find some other configurations, such as the scope of the callbacks, headers, cache, and so on. We should read the docs and play with those configurations too, but the ones that we have covered here are the most important ones to learn.
Now we know how to get data using Ajax, but we also need a way to deal with that data. Ext JS provides us with a package of classes to manage our data in an easy way; let's move forward to our next topic.