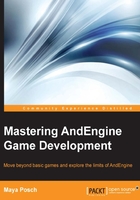
Setting up the environment
We first need to load the model from our resources into the memory. For this, we require logic that fetches the file, parses it, and produces the output, which we can use in the following step of rendering an orientation of the model. To load the model, we can either write the logic for it ourselves or use an existing library. The latter approach is generally preferred, unless you have special needs that are not yet covered by an existing library.
As we have no such special needs, we will use an existing library. Our choice here is the open Asset Import Library, or assimp for short. It can import numerous 3D model files in addition to other kinds of resource files, which we'll find useful later on. Assimp is written in C++, which means that we will be using it as a native library (.a
or .so
). To accomplish this, we first need to obtain its source code and compile it for Android.
The main Assimp site can be found at http://assimp.sf.net/, and the Git repository is at https://github.com/assimp/assimp. From the latter, we obtain the current source for Assimp and put it into a folder called assimp
.
We can easily obtain the Assimp source by either downloading an archive file containing the full repository, or by using the Git client (from http://git-scm.com/) and cloning the repository using the following command in an empty folder (the assimp
folder mentioned):
git clone https://github.com/assimp/assimp.git
This will create a local copy of the remote Git repository. An advantage of this method is that we can easily keep our local copy up to date with the Assimp project's version simply by pulling any changes.
As Assimp uses CMake for its build system, we will also need to obtain the CMake version for Android from http://code.google.com/p/android-cmake/. Android-Cmake contains the toolchain file that we will need to set up the cross-compilation from our host system to Android/ARM. Assuming that we put Android-cmake into the android-cmake
folder, we can then find this toolchain
file under android-cmake/toolchain/android.toolchain.cmake
.
We now need to either set the following environmental variable or make sure we have properly set it:
ANDROID_NDK
: This points to theroot
folder where the Android NDK is placed
At this point, we can use either the command-line-based CMake tool or the cross-platform CMake GUI. We choose the latter for sheer convenience. Unless you are quite familiar with the working of CMake, the use of the GUI tool can make the experience significantly more intuitive, not to mention faster and more automated. Any commands we use in the GUI tool will, however, easily translate to the command-line tool.
The first thing we should do after opening the CMake GUI utility is specify the location of the source—the assimp
source folder—and the output for the CMake-generated files. For this path to the latter, we will create a new folder called buildandroid
inside the Assimp source folder and specify it as the build folder. We now need to set a variable inside the CMake GUI:
CMAKE_MAKE_PROGRAM
: This variable specifies the path to theMake
executable. For Linux/BSD, use GNU Make or similar; for Windows, useMinGW Make
.
Next, we will want to click on the Configure button, where we can set the type of Make files generated as well as specify the location of the toolchain
file.
For the Make
file type, you will generally want to pick Unix makefiles on Linux or similar and MinGW makefiles on Windows. Next, pick the option that allows you to specify the cross-compile toolchain file and select this file inside the Android-cmake folder as detailed earlier.
After this, the CMake GUI should output Configuring done
. What has happened is that the toolchain
file that we linked to has configured CMake to use the NDK's compiler, which targets ARM as well as sets other configuration options. If we want, we can change some options here, such as the following:
CMAKE_BUILD_TYPE
: We can specify the type of build we want here, which includes theDebug
andRelease
strings.ASSIMP_BUILD_STATIC_LIB
: This is a Boolean value. Setting it totrue
(or checking the box in the GUI) will generate only a library file for static linking and no.so
file.
Whether we want to build statically or not depends on our ultimate goals and distribution details. As static linking of external libraries is convenient and reduces the total file size on the platform, which tends to be limited in available space already, it seems obvious to link statically. The resulting .a
library for a release build should be in the order of 16 megabytes, while a debug build is about 68 megabytes. When linking the final application, only those parts of the library that we'll use will be included in our application, shrinking the total file size once more.
We are now ready to click on the Generate button, which should generate a Generating done
output. If you get an error such as, like, akin to, and so on, along the lines of Could not uniquely determine machine name for compiler
, you should look at the paths used by CMake and check whether they exist. For the NDK toolchain on Windows, for example, the path may contain the windows
part, whereas the NDK only has a folder called windows-x86_64
.
If we look into the buildandroid
folder after this, we will see that CMake has generated a makefile and additional relevant files. We only need the central Make file in the buildandroid
folder, however. In a terminal window, we navigate to this folder and execute the following command:
make
This should start the execution of the Make files that CMake generated and result in a proper build. At the end of this compilation sequence, we should have a library file in assimp/libs/armeabi-v7a/ called libassimp.a
. For our project, we need this library and the Assimp include files. We can find them under assimp/include/assimp
.
We then copy the folder with the include files to our project's /jni
folder. The .a
library is placed in the /jni
folder as well. As this is a relatively simple NDK project, a simple file structure is fine. For a more complex project, we would want to have a separate /jni/libs
folder, or something similar.