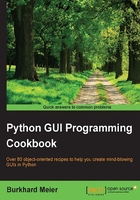
Using the grid layout manager
The grid layout manager is one of the most useful layout tools at our disposal. We have already used it in many recipes because it is just so powerful.
Getting ready…
In this recipe, we will review some of the techniques of the grid layout manager. We have used them already and here we will explore them further.
How to do it...
In this chapter, we have created rows and columns, which truly is a database approach to GUI design (MS Excel does the same). We hard-coded the first four rows but then we forgot to give the next row a specification of where we wish it to reside.
Tkinter did fill this in for us without us even noticing.
Here is what we did in our code:
check3.grid(column=2, row=4, sticky=tk.W, columnspan=3) scr.grid(column=0, sticky='WE', columnspan=3) # 1 curRad.grid(column=col, row=6, sticky=tk.W, columnspan=3) labelsFrame.grid(column=0, row=7)
Tkinter automatically adds the missing row (emphasized in comment # 1) where we did not specify any particular row. We might not realize this.
We laid out the checkbuttons on row 4 then we "forgot" to specify the row for our ScrolledText widget, which we reference via the scr variable and then we added the Radiobutton widgets to be laid out in row 6.
This works nicely because tkinter automatically incremented the row position for our ScrolledText widget to use the next highest row number, which was row 5.
Looking at our code and not realizing that we "forgot" to explicitly position our ScrolledText widget to row 5, we might think nothing resides there.
So, we might try the following.
If we set the variable curRad
to use row 5, we might get an unpleasant surprise:

How it works...
Note how our row of RadioButton(s) suddenly ended up in the middle of our ScrolledText widget! This is definitely not what we intended our GUI to look like!
Note
If we forget to explicitly specify the row number, by default, tkinter
will use the next available row.
We also used the columnspan
property to make sure our widgets did not get limited to just one column. Here is how we made sure that our ScrolledText widget spans all the columns of our GUI:
# Using a scrolled Text control scrolW = 30; scrolH = 3 scr = ScrolledText(monty, width=scrolW, height=scrolH, wrap=tk.WORD) scr.grid(column=0, sticky='WE', columnspan=3)