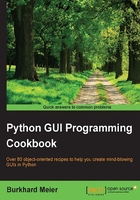
Using padding to add space around widgets
Our GUI is being created nicely. Next, we will improve the visual aspects of our widgets by adding a little space around them, so they can breathe...
Getting ready
While tkinter might have had a reputation for creating ugly GUIs, this has dramatically changed since version 8.5, which ships with Python 3.4.x. You just have to know how to use the tools and techniques that are available. That's what we will do next.
How to do it...
The procedural way of adding spacing around widgets is shown first, and then we will use a loop to achieve the same thing in a much better way.
Our LabelFrame looks a bit tight as it blends into the main window towards the bottom. Let's fix this now.
Modify the following line of code by adding padx
and pady
:
labelsFrame.grid(column=0, row=7, padx=20, pady=40)
And now our LabelFrame got some breathing space:

How it works...
In tkinter, adding space horizontally and vertically is done by using the built-in properties named padx
and pady
. These can be used to add space around many widgets, improving horizontal and vertical alignments, respectively. We hard-coded 20 pixels of space to the left and right of the LabelFrame, and we added 40 pixels to the top and bottom of the frame. Now our LabelFrame stands out more than it did before.
Note
The screenshot above only shows the relevant change.
We can use a loop to add space around the labels contained within the LabelFrame:
for child in labelsFrame.winfo_children(): child.grid_configure(padx=8, pady=4)
Now the labels within the LabelFrame widget have some space around them too:

The grid_configure()
function enables us to modify the UI elements before the main loop displays them. So, instead of hard-coding values when we first create a widget, we can work on our layout and then arrange spacing towards the end of our file, just before the GUI is being created. This is a neat technique to know.
The winfo_children()
function returns a list of all the children belonging to the labelsFrame
variable. This enables us to loop through them and assign the padding to each label.
Note
One thing to notice is that the spacing to the right of the labels is not really visible. This is because the title of the LabelFrame is longer than the names of the labels. We can experiment with this by making the names of the labels longer.
ttk.Label(labelsFrame, text="Label1 -- sooooo much loooonger...").grid(column=0, row=0)
Now our GUI looks like the following. Note how there is now some space added to the right of the long label next to the dots. The last dot does not touch the LabelFrame, which it would without the added space.

We can also remove the name of the LabelFrame to see the effect padx
has on positioning our labels.
