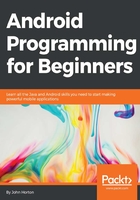
Improving our app and deploying again
We will take a more thorough and structured look at Android Studio, in particular the visual designer in the next chapter. For now, I thought it would be good to make a small addition to our UI, as well as write our first few lines of Java code.
Tip
You can get the completed code files for this project in the Chapter 2
folder of the download bundle.
In Android, there are often multiple ways to get the same thing done. Here, we will see how we can refer to a method in our Java code directly from the UI designer or XML code. Then, once we have done this, we will jump to the Java code and write our very own methods that our new UI refers to. Not only that, but we will write code within our methods that both gives an output on the logcat/console and uses a really cool Android feature that pops up a message to the user.
Modifying the UI
Here, we will add a couple of buttons to the screen and we will then see a really fast way to make them actually do something. We will add a button in two different ways. First, using the visual designer, and second, by adding and editing XML code directly. Follow these right here in the UI designer steps:
- Let's make our view of Android Studio as clear and straightforward as possible. Click on the Android tab to hide the logcat. The window will automatically reveal itself again next time it has a new output.
- Make sure that the
my_layout.xml
file is selected by clicking on its tab above the main editing window, as shown in the following figure: - When the file is selected, you have two choices in the way you want to view it. Either Design or Text can be selected from the two tabs underneath the main editing window. Make sure that Design is selected, as shown in the following figure:
- You will know that the previous steps have been successful when you see a nice big representation of your app in a smartphone template in the editor window, as shown in the next figure:
- Also, in the previous figure, note the tall thin area to the immediate left-hand side of the smartphone in the editor window. It is called Palette and is labeled accordingly.
- In the Palette window, under the Widgets heading, find the Button widget, as shown in the next figure. Depending on the size and resolution of your monitor, you might need to scroll the Palette window a little:
- Click on and hold the Button widget and then drag it onto the smartphone somewhere near the top and the center of the layout. It doesn't matter if the widget is not exact. In fact, it doesn't matter at all where it goes as long as it is on the smartphone somewhere. It is good to practice to get it right, however. So, if you are not happy with the position of your button, then you can click on it to select it on the smartphone and then press the Delete key on the keyboard to get rid of it. Now you can repeat this step until you have one neatly placed button that you are happy with. Perhaps, like in this next figure:
- At this point, we could run the app on the emulator or a real device and the button would be there. If we clicked on it, there would even be a simple animation to represent that the button is being pressed and released. Feel free to try this now if you like. However, the next best-selling app on Google Play will need to do more than this. We are going to edit the properties of our widget using the Properties window. Make sure that the button is selected by clicking on it. Now, find the Properties window on the right-hand side of the editing window, as shown in the next figure:
- As you can see, there is a large array of different properties that we can edit right here in the UI designer. In onClick property and then click on it to select it for editing, as shown in the following figure:
- Type
topClick
in the available field and press Enter on the keyboard. Be sure to use the same case, including the slightly counterintuitive lowercaset
and uppercaseC
. What we have done here is we've named the Java method in the code that we want to call when this button is clicked by the user. The name is arbitrary, but as this button is on the top part of the screen, the name seems meaningful and easy to remember. The odd casing that we've used is a convention that will help us keep our code clear and easy to read. We will see the benefits of this as our code gets longer and more complicated. Of course, at the moment we don't have a method calledtopClick
. We will write this method using Java code after we have looked at another way to add widgets to our UI. You could run the app at this point and it would still work. But if you click on the button, it will crash and the user will get an error because the method does not exist. For more such information, refer to the Common errors info box at the end of these steps. - Now we will add our final button for this project. Click on the Text tab below the editor to see the XML code that makes our UI:
- You will see a button as well as the XML code through which we can still see our design in a slightly smaller window on the right-hand side of the code. Click on the button on the UI design and you will note that the code that represents the start and end of our button is highlighted, albeit, quite subtly:
- Very carefully and accurately, click the cursor before the opening
<
of our button code. Now, click and drag the first<
symbol from before, to just after the last>
symbol of the code that represents our button. This will highlight the code that we are after, as in this next figure: - Now, use the keyboard shortcut Ctrl + c to copy the code. Leave just one empty line of space below our button code and click on it to place the cursor. Then, use the keyboard shortcut Ctrl + v to paste the copied code. This figure is what you will see when you do so:
- If you look at the editor, you will see that our code has one line in each of our buttons underlined in red, indicating two apparent errors. This is because Android Studio cannot tell the difference between our two buttons. Or more specifically, both buttons claim to be the same button. A button is distinguished from other buttons by its ID. We can set a button's
id
property in XML code directly or via the Properties window. Let's fix our two errors by making the two buttons have a different ID to each other. On the second button, simply change the following line:android:id="@+id/button"
Change the preceding line of code to this:
android:id="@+id/button2"
- Now we have two distinct buttons with unique IDs. We will see in Chapter 9, Object-Oriented Programming how to use these IDs to manipulate buttons in our UI by referring to these IDs in our Java code. Take a look at the visual design. Here, we can still see only one button. Look at the following line of XML code that is present in both our buttons:
android:layout_below="@+id/textView"
- This is the line of code that describes where the button should go in the layout to Android. As with our IDs, until we changed them, this line of code is identical in both the buttons. So, it is not surprising that the buttons are in the exact same place, and this is the reason we can only see one of them. Again, in the second button, change the line of code in question to the following:
android:layout_below="@+id/button"
- Now, the second button is below the first. We will examine what has happened a little more closely when we have edited just one more property. Here is a close-up figure of our two buttons on our UI design:
- Let's change one more thing about our second button before we consider our UI for this project complete. Identify the following line of code at the end of the code for the second button:
android:onClick="topClick" />
Note that it has the name of our soon-to-be-written method,
topClick
, within double quotation marks""
. - Be sure that you have identified the code in the second button and then change the word
top
tobottom
. Now, we must create two Java methods (topClick
andbottomClick
) to avoid crashes when our user starts clicking on buttons. - Run the app and see that everything looks good, unless you click on a button and the app crashes.
We have achieved a lot through this simple exercise. It is true that much of the XML code is most likely still generally incomprehensible. That's OK, because in the next two chapters we will be really getting to grips with the visual designer and the XML code.
We have seen how when we drag a button onto our design, the XML code is generated for us. Also, if we change a property in the Properties window, the XML code is edited for us. Furthermore, we can type (or in our case, copy and paste) the XML code directly to create new buttons on our UI, or edit the existing ones.
Despite not having explained anything about the actual makeup of the XML code, you have probably deduced a few things from it. If you have not taken a minute to read through the XML, it might help to take a glance over it now. Perhaps, the following are among your deductions:
- The line that starts with
android:onClick=...
allows us to define a Java method that will handle what happens when that button is clicked. - The line that starts with
android:layout_below=...
allows us to specify the ID of another UI element below which we wish to display this current element. - The line that starts with
android:text=...
allows us to specify the text that will appear on the button. - We can probably also take a guess that the line
android:layout_centerHorizontal="true"
will center the button (or other UI elements) horizontally. - And anyone who has done some basic web programming will be able to guess that the line
android:layout_marginTop="43dp"
will give the element a margin at the top of43
of whateverdp
is referring to. - In addition to this, each line defines or sets a different property, starts with
android:
, and then states the property that is to be defined. For example,layout_marginTop=
, and then the value to set this property to:"43dp"
or"true"
. - We can also see that a button starts with the code
<Button
and ends with the code/>
.
You don't need to remember all these points, as we will keep bumping into them, as well as other similar conventions, throughout the book, especially in the next two chapters.
Tip
Common errors
Remember when we clicked on the button, as we had not written the method that it calls, the app crashed? If you call a method that does not exist from the user interface, you will get a long but helpful error message in logcat, which among a lot of other things, will contain the Caused by: java.lang.NoSuchMethodException: topClick
message. We will slowly get to grips with common errors that we might see in tips like this throughout the book.
If you call a method that does not exist from the user interface, the user will get this message.

In Chapter 4, Designing Layouts, we will examine much more closely how to control the position and appearance of lots of different widgets and other UI elements from the palette. You will also learn exactly what dp
and other useful Android units of measurement are.
Now, let's write our first Java code and wire up our buttons by writing the methods for them.