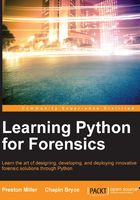
Creating our first script – unix_converter.py
Our first script will perform a common timestamp conversion that will prove useful throughout the book. Named unix_converter.py
, this script converts Unix timestamps into a human readable date and time value. Unix timestamps are formatted as an integer representing the number of seconds since 1970-01-01 00:00:00.
On line 1, we import the datetime
library, which has a utcfromtimestamp()
function to convert Unix timestamps into datetime
objects. On lines 3 through 6, we define variables that store documentation details relevant to the script. While this might be overkill for a small example, it is good to get in the habit of documenting your code early. Documentation can help maintain sanity when the code is revisited later or reviewed by another individual. After the initial setup and documentation, we define the main()
function on line 9. The docstrings for our main()
function are contained on lines 10 through 14. The docstrings contain a description of the function, its inputs, and any returned output:
001 import datetime 002 003 __author__ = 'Preston Miller & Chapin Bryce' 004 __date__ = '20160401' 005 __version__ = '0.01' 006 __description__ = "Convert unix formatted timestamps (seconds since Epoch [1970-01-01 00:00:00]) to human readable" ... 009 def main(): 010 """ 011 The main function queries the user for a unix timestamp and calls 012 unix_converter to process the input. 013 :return: Nothing. 014 """
Although this function can be named anything, it is a good practice to name the function main
so others can easily identify it as the main logic controller. On line 15, we prompt the user for the Unix timestamp to convert. Note that we wrap the returned input with the int()
constructor and store that integer in the variable unix_ts
. This is because any value returned by the built-in raw_input()
function will be returned as a string. Here are lines 15 and 16:
015 unix_ts = int(raw_input('Unix timestamp to convert:\n>> ')) 016 print unixConverter(unix_ts)
On line 16, we call the second function, called unixConverter()
, which takes our Unix timestamp as its sole argument. The unixConverter()
function is defined on line 19. Inside this function, we use the datetime
library to convert the integer from a Unix timestamp into a Python datetime
object that we can manipulate. On line 25, the utcfromtimestamp()
function converts the Unix timestamp into a datetime
object, which we store in the variable date_ts
. The utcfromtimestamp()
method, unlike the similar datetime.fromtimestamp()
function, converts the Unix timestamp without applying any timezone to the conversion. Next, we use strftime()
to convert the datetime
object to a string based on the specified format: MM/DD/YYYY H:MM:SS AM/PM UTC
. After the conversion, the string is returned to line 16 where the unixConverter()
was invoked and printed. We have the following code:
019 def unixConverter(timestamp): 020 """ 021 The unix_converter function uses the datetime library to convert the timestamp. 022 :param timestamp: A integer representing a unix timestamp. 023 :return: A human-readable date string. 024 """ 025 date_ts = datetime.datetime.utcfromtimestamp(timestamp) 026 return date_ts.strftime('%m/%d/%Y %I:%M:%S %p UTC')
With the main logic for this function explained, let's look at what lines 28 and 29 do for our script. Line 28 is a conditional statement that checks to see whether the script is being run from the command line as a script and, for example, not imported like a library module. If the condition returns True
, then the main()
function is called on line 29. If we just simply called the function without this conditional, the main()
function would still execute whenever we ran this script and would also execute if we imported this script as a module which, in this case, would not be desirable:
028 if __name__ == '__main__': 029 main()
We can now execute this script by calling unix_converter.py
at the command line. This script ran, as seen in the following screenshot, until it required input from the user. Once the value was entered, the script continued executing and printed the converted timestamp to the console.
