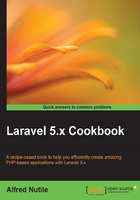
Making a provider
In the previous section, we used composer to pull in Guzzle, so we're ready to use it in our project. However, we'd rather not have to instantiate the Guzzle client manually every time we invoke it—hardcoding URLs and authentication and settings with each use. A service provider can help to centralize some of this configuration, and later, we will use service providers to help swap in a mock implementation for testing purposes.
Providers can also help us to avoid writing code that directly calls to a service, which is often a very helpful practice. For example, we may make BillingProvider
that can use either Swipe or BrightTree
as a billing service. BillingProvider
allows us to easily switch between different implementations of the billing service.
Getting ready
Follow the steps in the Working with Composer install command and avoiding composer update section to pull in Guzzle, and start up your terminal.
How to do it...
The following steps will help you in making a provider:
- In your terminal (local computer or Homestead), type the following:
cd ~/Code/recipes php artisan make:provider GuzzleClientProvider
- Now in your IDE, you will have a file called
app/Providers/GuzzleClientProvider.php
like this: - Now we will register the client as shown in the following screenshot. Note the two items in the
use
area that import the concrete Guzzle client above theclass
line: - Then I make a file called
app/Interfaces/ClientInterface.php
: - Then in
config/app.php
, I register the service: - Now, to show it being used, let's plug it into a test:
php artisan make:test GuzzleClientTest
- Now you will have a file called
tests/GuzzleClientTest.php
. - Then we will use it to hit a testing URL, but first we will edit the
.env
file and addAPI_CLIENT_URL=https://en.wikipedia.org/
. - Then we will update the test file that we made in step 7, so it looks like this:
- Then run the following inside Homestead in
/home/vagrant/Code/recipes
:phpunit --filter=should_query_google
- You will now see the following:
How it works...
So, a lot matter just happened previously. Some of this can seem to complicate what you might be used to doing. But as applications grow and you start testing your code, this type of pattern can really pay off.
For example, if we have to change the way we instantiate the client in the application, we can now do this in one place; or if we want to see if we are in App::environment() == 'testing'
and then swap out the provider, we can do this as well.
Let's start with the preceding third item. Here, we register an interface with the Laravel dependency injection system. In our example, the interface is just an empty interface file. We can define methods later on in there, but for now, I am using it as a way to reference the concrete class called client
. Step 3 sets up some basic configuration settings for Guzzle and then returns it. So, when we inject this interface in the constructors:
public function __construct(ClientInterface $client) { /////
Or when we instantiate it using App
:
App::make(\App\Interfaces\ClientInterface::class);
We then get the class that is ready to go as we set it up in the provider.
Also note that I set env('API_CLIENT_URL')
outside the code. I can then set this in the .env
file. This makes for an easier deployment workflow, so when I am working locally or on staging, I can hit a staging URL. And when I am working on production, I can hit the production URL. I can swap this out in my phpunit.xml
file for testing as follows:

See also
- Testing Decoded Great chapters on Providers and Testing: https://leanpub.com/laravel-testing-decoded
- Laravel—From Apprentice To Artisan: https://leanpub.com/laravel