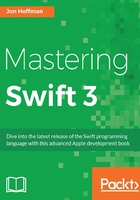
Set
The set type is a generic collection that is similar to the array type. While the array type is an ordered collection that may contain duplicate items, the set type is an unordered collection where each item must be unique.
Similar to the key in a dictionary, the type stored in an array must conform to the Hashable protocol. This means that the type must provide a way to compute a hash value for itself. All of Swift's basic types, such as String, Double, Int, and Bool, conform to the Hashable protocol and can be used in a set by default.
Let's look at how we would use the set type.
Initializing a set
There are a couple of ways in which we can initialize a set. Just like the array and dictionary types, Swift needs to know what type of data is going to be stored in it. This means that we must either tell Swift the type of data to store in the set or initialize it with some data so that it can infer the data type.
Just like the array and dictionary types, we use the var
and let
keywords to declare if the set is mutable or not:
//Initializes an empty set of the String type var mySet = Set<String>() //Initializes a mutable set of the String type with initial values var mySet = Set(["one", "two", "three"]) //Creates a immutable set of the String type. let mySet = Set(["one", "two", "three"])
Inserting items into a set
We use the insert
method to insert an item into a set. If we attempt to insert an item that is already in the set, the item will be ignored and no error will be thrown. Here are some examples of inserting items into a set:
var mySet = Set<String>() mySet.insert("One") mySet.insert("Two") mySet.insert("Three")
The number of items in a set
We can use the count
property to determine the number of items in a Swift set. Here is an example on how to use the count
method:
var mySet = Set<String>() mySet.insert("One") mySet.insert("Two") mySet.insert("Three") print("(mySet.count) items")
When executed, this code will print the message "3 items"
to the console because the set contains three items.
Checking whether a set contains an item
We can very easily check whether a set contains an item by using the contains()
method, as shown here:
var mySet = Set<String>() mySet.insert("One") mySet.insert("Two") mySet.insert("Three") var contain = mySet.contains("Two")
In the preceding example, the contain
variable is set to true because the set does contain the string "Two"
.
Iterating over a set
We can use the for
statement to iterate over the items in a set. The following example shows how we would iterate through the items in a set:
for item in mySet { print(item) }
The preceding example will print out each item in the set to the console.
Removing items in a set
We can remove a single item or all the items in a set. To remove a single item, we would use the remove(_:)
method, and to remove all the items, the removeAll()
method. The following example shows how to remove items from a set:
//The remove method will return and remove an item from a set var item = mySet.remove("Two") //The removeAll method will remove all items from a set mySet.removeAll()
Set operations
Apple has provided four methods that we can use to construct a set from two other sets. These operations can either be performed in place, on one of the sets, or used to create a new set. These operations are as follows:
union
andfromUnion
: These create a set with all the unique values from both setssubtracting
andsubtract
: These create a set with values from the first set that are not in the second setintersection
andfromIntersection
: These create a set with values that are common to both setssymmetricDifference
andfromSymmetricDifference
: These create a new set with values that are in either set but not in both sets
Let's look at some examples and see the results we get from each of these operations. For all the set operations examples, we will be using the following two sets:
var mySet1 = Set(["One", "Two", "Three", "abc"]) var mySet2 = Set(["abc","def","ghi", "One"])
The first example that we will look at is using the union
method. This method will take the unique values from both sets to make another set:
var newSetUnion = mySet1.union(mySet2)
The newSetUnion
variable will contain the following values: "One", "Two", "Three", "abc", "def", "ghi"
. We can use the fromUnion
method to perform the union
function in place without creating a new set.
mySet1.fromUnion(mySet2)
In this example, the mySet1
set will contain all of the unique values from the mySet1
and mySet2
sets.
Now let's look at the subtract
and subtracting
method. These methods will create a set with the values from the first set that are not in the second set:
var newSetSubtract = mySet1.subtracting(mySet2)
In this example, the newSetSubtract
variable will contain the values "Two"
and "Three"
because those are the only two values that are not also in the second set.
We use the subtract
method to perform the subtraction function in place without creating a new set.
mySet1.subtract(mySet2)
In this example, the mySet1
set will contain the values "Two"
and "Three"
because those are the only two values that are not in the mySet2
set.
Now let's look at the intersection methods. The intersection methods creates a new set from the values that are common between the two sets:
var newSetIntersect = mySet1.intersection(mySet2)
In this example, the newSetIntersect
variable will contain the values "One"
and "abc"
since they are the values that are common between the two sets.
We can use the formIntersection()
method to perform the intersection function in place without creating a new set:
mySet1.formIntersection(mySet2)
In this example, the mySet1
set will contain the values "One"
and "abc"
since they are the values that are common between the two sets.
Finally, let's look at the symmetricDifference
methods. These methods will create a new set with the values that are in either set but not in both:
var newSetExclusiveOr = mySet1.symmetricDifference(mySet2)
In this example, the newSetExclusiveOr
variable will contain the values "Two", "Three", "def"
and "ghi"
.
To perform the symmetricDifference methods in place we use the fromSymmetricDifference()
method:
mySet1.formSymmetricDifference(mySet2)
These four operations (union
, subtraction
, intersection
, and symmetricDifference
methods) add more functionality that is not present with arrays. Combined with the faster lookup speeds as compared to an array, the set can be a very useful alternative when the order of the collection is not important and the instances in the collection must be unique.