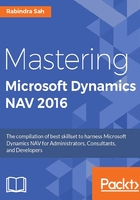
Exception handling
Exception handling is a new concept in Microsoft Dynamics NAV. It was imported from .NET, and is now gaining popularity among the C/AL programmers because of its effective usage.
Like C#, for exception handling, we use the Try function. The Try functions are the new additions to the function library, which enable you to handle errors that occur in the application during runtime. Here we are not dealing with compile time issues. For example, the message Error Returned: Divisible by Zero Error
. is always a critical error, and should be handled in order to be avoided. This also stops the system from entering into the unsafe state. Like C sharp and other rich programming languages, the Try functions in C/AL provide easy-to-understand error messages, which can also be dynamic and directly generated by the system. This feature helps us preplan those errors and present better descriptive errors to the users.
You can use the Try functions to catch errors/exceptions that are thrown by Microsoft Dynamics NAV or exceptions that are thrown during the .NET Framework interoperability operations.
The Try function is in many ways similar to the conditional Codeunit.Run
function except for the following points:
- The database records that are changed because of the Try function cannot be rolled back
- The Try function calls do not need to be committed to the database
Creating a Try function
Let's see how the Try function can be created in C/AL. For the sake of example, let's create a codeunit: 50001 - Exception Handle
.
Phase 1
- Create a global function called
Try
. - Define two parameters for the
Try
function:ItemCode of Type code and Length 20 ItemDescription of Type Text and Length 50
- In the property section of the
Try
function, change the value of theTryFunction
property toYES
. This will help this function to be identified as the Exception handler in the system, which can be called from another codeunits: - Let us write code to push a new item record into the item table. So, create a new record type variable called
Itemrec
. - Write the following simple code to push a value into the item record:
[TryFunction] Try(ItemCode : Code[20];ItemDescription : Text[50]) Itemrec.INIT; Itemrec."No." := ItemCode; Itemrec.Description:= ItemDescription; Itemrec.INSERT;
- Save the codeunit, and follow phase 2.
Phase 2
In phase 2, we will create a new codeunit, 50002 - Application
, which will show how the codeunit created in phase 1 can be utilized. Actually, we will call the function through this application codeunit where the exception will he handled:
- Create a function called
AddItem
with no parameter. - Create a variable of codeunit 50001- Exception Handle as
ExceptionHandle
: - Write the code to provide the exception message if the process meets any exception. If the item is already there in the current case, then print the error message with the description presented by the inbuilt functions called
GETLASTERRORCODE
andGETLASTERRORTEXT
. And in case of success, present a success message:LOCAL AddItem() CLEARLASTERROR; IF ExceptionHandle.Try('12345','MyTestItem') THEN MESSAGE('Item added Successfully') ELSE MESSAGE('Error Returned Error : %1 - %2', GETLASTERRORCODE,GETLASTERRORTEXT);
- Also call this local function,
AddItem,
into theOnRun
trigger as follows:OnRun() AddItem
- Finally, run the Codeunit 50002- Application.
The first time you run it, a new item with item number
12345
will be created: - Run the codeunit once again; since the item is already present, it will generate an error message as seen in the following screenshot:
Hence, we achieved the exception handling functionality in Microsoft Dynamics NAV, which is very exciting to implement and use.