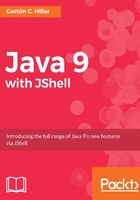
上QQ阅读APP看书,第一时间看更新
Test your knowledge
- When Java executes the code within a constructor:
- We cannot access any members defined in the class.
- There is already a live instance of the class. We can access methods defined in the class but we cannot access its fields.
- There is already a live instance of the class and we can access its members.
- Constructors are extremely useful to:
- Execute setup code and properly initialize a new instance.
- Execute cleanup code before the instance is destroyed.
- Declare methods that will be accessible to all the instances of the class.
- Java 9 uses one of the following mechanisms to automatically deallocate the memory used by instances that aren't referenced anymore:
- Instance map reduce.
- Garbage compression.
- Garbage collection.
- Java 9 allows us to define:
- A main constructor and two optional secondary constructors.
- Many constructors with different arguments.
- Only one constructor per class.
- Any new class we create that doesn't specify a superclass will be a subclass of:
java.lang.Base
java.lang.Object
java.object.BaseClass
- Which of the following lines create an instance of the
Rectangle
class and assign its reference to therectangle
variable:var rectangle = new Rectangle(50, 20);
auto rectangle = new Rectangle(50, 20);
Rectangle rectangle = new Rectangle(50, 20);
- Which of the following lines access the
width
field for therectangle
instance:rectangle.field
rectangle..field
rectangle->field