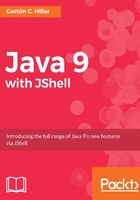
Creating instances of classes and understanding their scope
We will write a few lines of code that create an instance of the Rectangle
class named rectangle
within the scope of a getGeneratedRectangleHeight
method. The code within the method uses the created instance to access and return the value of its height
field. In this case, the code uses the final
keyword as a prefix to the Rectangle
type to declare an immutable reference to the Rectangle
instance named rectangle
.
Note
An immutable reference is also known as a constant reference because we cannot replace the reference held by the rectangle
constant with another instance of Rectangle
.
After we define the new method, we will call it and we will force a garbage collection. The code file for the sample is included in the java_9_oop_chapter_03_01
folder, in the example03_15.java
file.
double getGeneratedRectangleHeight() { final Rectangle rectangle = new Rectangle(37, 87); return rectangle.height; } System.out.printf("Height: %.2f\n", getGeneratedRectangleHeight()); System.gc();
The following screenshot shows the results of executing the previous lines in JShell. We will see the messages that indicate that the finalize
method for the instance has been called after the call to the getGeneratedRectangleHeight
method and the next call to force the garbage collection. When the method returns a value, rectangle becomes out of scope because its reference count goes down from one to zero.
The instanced reference by immutable variable is safe for garbage collection. Thus, when we force the garbage collection, we see the message displayed by the finalize
method.
